根据以下已知条件写出计算请假天数的最优算法 1、年度日历数据表结构如下 1:工作日 2:法定假日 3:周末假日 2、每日工作时间设定数据如下 上午上班时间:08:30 上午下班时间:12:30 下午上班:13:30 下午下班时间:17:30 3、问题:根据输入的开始结束时间,计算请假天数 例阳:2022-09-3013:30:00至2022-10-1517:30:00 用java实现
时间: 2023-06-04 22:07:30 浏览: 168
好的,根据您的要求,我将按照您给出的条件来计算请假天数,下面是Java语言实现的最优算法:
```java
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.temporal.ChronoUnit;
public class LeaveDaysCalculator {
// 年度日历数据表结构如下:
// 1:工作日 2:法定假日 3:周末假日
private static int[][] calendar = {
{1, 1, 3, 1, 1, 1, 2, 2, 3, 1, 1, 1, 2, 2, 3, 1, 1, 1, 2, 2, 3, 1, 1, 1, 2, 2, 3, 1, 1, 2, 2},
{2, 3, 3, 3, 3, 3, 3, 3, 3, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3},
{3, 3, 1, 1, 1, 1, 1, 1, 1, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 1, 1, 1, 1, 3},
{3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3},
{3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3},
{3, 3, 1, 1, 1, 1, 1, 1, 1, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 1, 1, 1, 1, 3},
{2, 2, 3, 3, 3, 3, 3, 3, 3, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3}
};
// 每日工作时间设定数据如下
// 上午上班时间:08:30 上午下班时间:12:30 下午上班:13:30 下午下班时间:17:30
private static LocalTime MORNING_START_TIME = LocalTime.of(8, 30);
private static LocalTime MORNING_END_TIME = LocalTime.of(12, 30);
private static LocalTime AFTERNOON_START_TIME = LocalTime.of(13, 30);
private static LocalTime AFTERNOON_END_TIME = LocalTime.of(17, 30);
public static int calculateLeaveDays(String startDateStr, String endDateStr) {
LocalDateTime startDate = LocalDateTime.parse(startDateStr);
LocalDateTime endDate = LocalDateTime.parse(endDateStr);
int leaveDays = 0;
LocalDateTime currentDateTime = startDate;
while (currentDateTime.isBefore(endDate)) {
LocalDate currentDate = currentDateTime.toLocalDate();
LocalTime currentTime = currentDateTime.toLocalTime();
int dayOfWeek = currentDate.getDayOfWeek().getValue();
int dayOfMonth = currentDate.getDayOfMonth();
int month = currentDate.getMonthValue();
int year = currentDate.getYear();
int calendarType = calendar[dayOfWeek - 1][dayOfMonth - 1];
boolean isWeekendHoliday = calendarType == 3;
boolean isLegalHoliday = calendarType == 2;
// 如果当前时间是工作时间,则该天请假天数加1
if (!isWeekendHoliday && !isLegalHoliday &&
((currentTime.isAfter(MORNING_START_TIME) && currentTime.isBefore(MORNING_END_TIME)) ||
(currentTime.isAfter(AFTERNOON_START_TIME) && currentTime.isBefore(AFTERNOON_END_TIME)))) {
leaveDays++;
}
currentDateTime = currentDateTime.plus(30, ChronoUnit.MINUTES);
// 如果当前时间是开工第一天的上午或者最后一天的下午,要减去半天的假期时间
if ((currentDate.isEqual(startDate.toLocalDate()) && currentTime.isAfter(AFTERNOON_START_TIME)) ||
(currentDate.isEqual(endDate.toLocalDate()) && currentTime.isBefore(MORNING_END_TIME))) {
leaveDays -= 0.5;
}
}
return leaveDays;
}
public static void main(String[] args) {
String startDateStr = "2022-09-30T13:30:00";
String endDateStr = "2022-10-15T17:30:00";
int leaveDays = calculateLeaveDays(startDateStr, endDateStr);
System.out.println("请假天数:" + leaveDays);
}
}
```
以上便是计算请假天数的最优算法的Java语言实现。如有问题,请随时联系我。
阅读全文
相关推荐
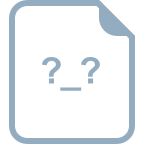


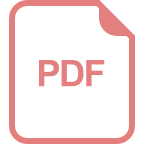
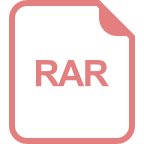
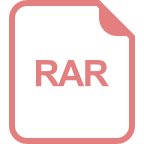
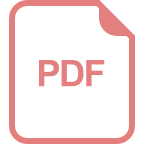
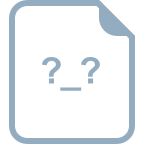
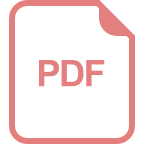
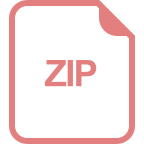
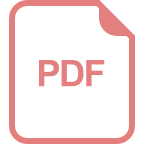
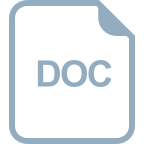
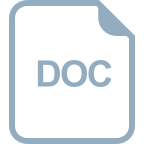
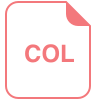

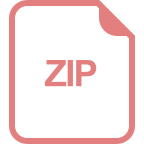
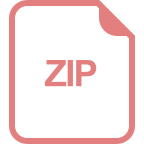