我想在adaboost中将它的基分类器换成ngboost,以iris为例给出代码
时间: 2023-11-22 11:55:34 浏览: 131
好的,首先需要安装 `ngboost` 和 `sklearn` 库,可以使用以下命令进行安装:
```
pip install ngboost
pip install sklearn
```
然后我们可以按照以下步骤来使用 `ngboost` 替换 `adaboost`:
1. 导入所需的库和数据集
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from ngboost import NGBoost
from ngboost.learners import default_tree_learner
from ngboost.distns import Categorical, Normal
# 加载 iris 数据集
iris = load_iris()
X, y = iris.data, iris.target
```
2. 将数据集划分为训练集和测试集
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
3. 定义 `ngboost` 模型并进行训练
```python
# 定义 ngboost 模型
ngb = NGBoost(natural_gradient=True, Dist=Categorical, Base=default_tree_learner)
# 训练 ngboost 模型
ngb.fit(X_train, y_train)
```
4. 使用训练好的模型进行预测,并计算准确率
```python
# 使用 ngboost 模型进行预测
y_pred = ngb.predict(X_test)
# 计算准确率
accuracy = np.sum(y_pred == y_test) / len(y_test)
print("Accuracy:", accuracy)
```
完整代码如下:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from ngboost import NGBoost
from ngboost.learners import default_tree_learner
from ngboost.distns import Categorical, Normal
# 加载 iris 数据集
iris = load_iris()
X, y = iris.data, iris.target
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义 ngboost 模型
ngb = NGBoost(natural_gradient=True, Dist=Categorical, Base=default_tree_learner)
# 训练 ngboost 模型
ngb.fit(X_train, y_train)
# 使用 ngboost 模型进行预测
y_pred = ngb.predict(X_test)
# 计算准确率
accuracy = np.sum(y_pred == y_test) / len(y_test)
print("Accuracy:", accuracy)
```
阅读全文
相关推荐
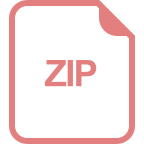


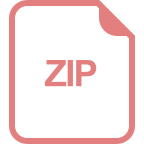
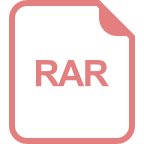
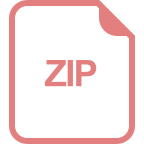
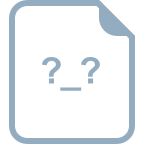
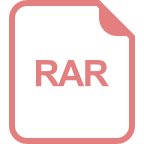
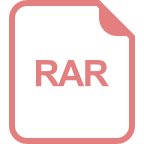
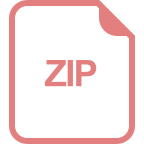
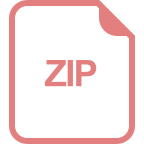
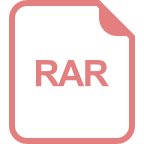
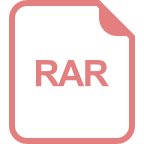
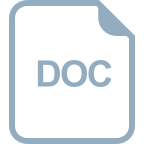
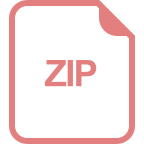