用RSA加密AES的密钥k,后用AES加密数据 用c语言实现
时间: 2024-05-06 10:18:59 浏览: 21
以下是用C语言实现RSA加密AES密钥和AES加密数据的代码,其中使用了OpenSSL库来实现RSA和AES加密算法:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/evp.h>
#include <openssl/rand.h>
#define AES_KEY_SIZE 256
#define AES_BLOCK_SIZE 128
#define RSA_KEY_SIZE 2048
int main() {
// 生成AES密钥
unsigned char aes_key[AES_KEY_SIZE/8];
if (RAND_bytes(aes_key, AES_KEY_SIZE/8) != 1) {
printf("Error generating AES key\n");
return 1;
}
// AES加密数据
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen(plaintext);
int ciphertext_len;
unsigned char iv[AES_BLOCK_SIZE/8];
RAND_bytes(iv, AES_BLOCK_SIZE/8);
unsigned char ciphertext[plaintext_len + AES_BLOCK_SIZE/8];
EVP_CIPHER_CTX *ctx;
if (!(ctx = EVP_CIPHER_CTX_new())) {
printf("Error creating AES context\n");
return 1;
}
if (EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, aes_key, iv) != 1) {
printf("Error initializing AES encryption\n");
return 1;
}
if (EVP_EncryptUpdate(ctx, ciphertext, &ciphertext_len, plaintext, plaintext_len) != 1) {
printf("Error encrypting AES data\n");
return 1;
}
int final_len;
if (EVP_EncryptFinal_ex(ctx, ciphertext + ciphertext_len, &final_len) != 1) {
printf("Error finalizing AES encryption\n");
return 1;
}
ciphertext_len += final_len;
EVP_CIPHER_CTX_free(ctx);
// RSA加密AES密钥
RSA *rsa_keypair = RSA_generate_key(RSA_KEY_SIZE, RSA_F4, NULL, NULL);
int rsa_len = RSA_size(rsa_keypair);
unsigned char rsa_plaintext[rsa_len];
memcpy(rsa_plaintext, aes_key, AES_KEY_SIZE/8);
unsigned char rsa_ciphertext[rsa_len];
if (RSA_public_encrypt(AES_KEY_SIZE/8, rsa_plaintext, rsa_ciphertext, rsa_keypair, RSA_PKCS1_OAEP_PADDING) == -1) {
printf("Error encrypting AES key with RSA\n");
return 1;
}
// 输出加密结果
printf("AES ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\nRSA ciphertext: ");
for (int i = 0; i < rsa_len; i++) {
printf("%02x", rsa_ciphertext[i]);
}
printf("\n");
RSA_free(rsa_keypair);
return 0;
}
```
在实际应用中,需要将RSA加密的AES密钥和AES加密的数据一起传输给接收方,接收方需要先使用RSA解密AES密钥,再使用解密后的AES密钥来解密AES加密的数据。
相关推荐
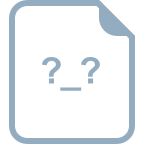














