用RSA加密AES的密钥k,后用AES加密数据c语言实现
时间: 2023-12-11 07:51:02 浏览: 170
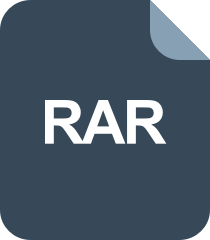
AES算法主要包括三个方面:轮变化、轮数和密钥扩展。本文以128为例,介绍算法的基本原理;结合C语言,实现高级数据加密算法AES
下面是用C语言实现RSA加密AES密钥k,然后用AES加密数据c的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/evp.h>
#include <openssl/aes.h>
#define KEY_LENGTH 2048
#define AES_BLOCK_SIZE 16
int main() {
// 生成RSA密钥对
RSA *rsa = RSA_generate_key(KEY_LENGTH, RSA_F4, NULL, NULL);
// 生成AES密钥
unsigned char aes_key[AES_BLOCK_SIZE];
if (!RAND_bytes(aes_key, AES_BLOCK_SIZE)) {
printf("Failed to generate AES key\n");
return 1;
}
// RSA加密AES密钥
int rsa_len = RSA_size(rsa);
unsigned char encrypted_key[rsa_len];
int encrypted_len = RSA_public_encrypt(AES_BLOCK_SIZE, aes_key, encrypted_key, rsa, RSA_PKCS1_PADDING);
if (encrypted_len == -1) {
printf("Failed to encrypt AES key\n");
return 1;
}
// 使用AES加密数据
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen(plaintext);
int ciphertext_len = 0;
unsigned char iv[AES_BLOCK_SIZE];
if (!RAND_bytes(iv, AES_BLOCK_SIZE)) {
printf("Failed to generate IV\n");
return 1;
}
unsigned char ciphertext[plaintext_len + AES_BLOCK_SIZE];
AES_KEY aes;
if (AES_set_encrypt_key(aes_key, AES_BLOCK_SIZE * 8, &aes) < 0) {
printf("Failed to set AES encryption key\n");
return 1;
}
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aes, iv, AES_ENCRYPT);
ciphertext_len = plaintext_len + AES_BLOCK_SIZE;
// 输出结果
printf("RSA encrypted AES key: ");
for (int i = 0; i < encrypted_len; i++) {
printf("%02x", encrypted_key[i]);
}
printf("\n");
printf("AES encrypted ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 释放内存
RSA_free(rsa);
return 0;
}
```
注意,上述代码需要引入OpenSSL库。可以使用以下命令进行编译:
```
gcc -o encrypt encrypt.c -lcrypto
```
其中,encrypt.c是包含上述代码的文件名,-lcrypto参数用于链接OpenSSL库。
需要注意的是,上述代码只是示例代码,实际使用时需要考虑更多的安全性问题,如密钥管理、数据完整性校验等。
阅读全文
相关推荐
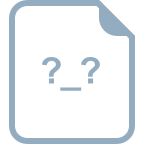

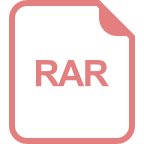
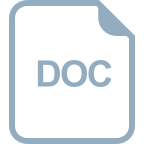
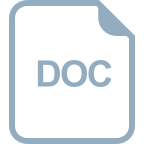
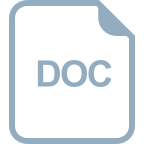
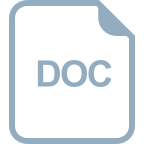
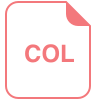
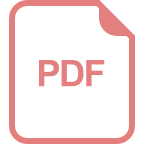
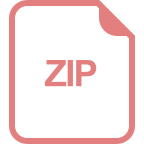
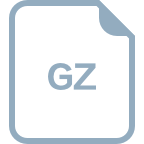
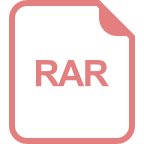
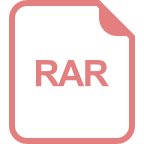
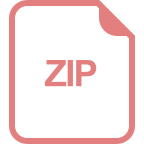
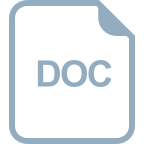
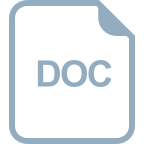
