用c++写一个民航订票系统,使用文件储存数据,不使用链表、容器,包含用户和管理两个类,实现订票、改签、退票、查询航班、增加航班、删除航班、修改航班、显示航班等功能,输出数据结构文件
时间: 2023-10-20 22:19:46 浏览: 35
以下是一个简单的用C++实现的民航订票系统,使用文件储存数据。注意,这只是一个示例,实际应用中还需要更多的功能和错误处理。
```c++
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
class User {
public:
User() {}
User(string name, int age, string id) {
this->name = name;
this->age = age;
this->id = id;
}
string name;
int age;
string id;
};
class Plane {
public:
Plane() {}
Plane(string id, string departure, string destination, string date, int ticketNum, int ticketPrice) {
this->id = id;
this->departure = departure;
this->destination = destination;
this->date = date;
this->ticketNum = ticketNum;
this->ticketPrice = ticketPrice;
}
string id;
string departure;
string destination;
string date;
int ticketNum;
int ticketPrice;
};
class Manager {
public:
Manager() {
user = NULL;
}
Manager(string name, string password) {
this->name = name;
this->password = password;
user = NULL;
}
bool login(string name, string password) {
if (this->name == name && this->password == password) {
return true;
}
return false;
}
void addUser(User* user) {
this->user = user;
}
User* getUser() {
return user;
}
void addPlane(Plane* plane) {
planes.push_back(plane);
}
void deletePlane(string id) {
for (int i = 0; i < planes.size(); i++) {
if (planes[i]->id == id) {
planes.erase(planes.begin() + i);
break;
}
}
}
void modifyPlane(string id, string departure, string destination, string date, int ticketNum, int ticketPrice) {
for (int i = 0; i < planes.size(); i++) {
if (planes[i]->id == id) {
planes[i]->departure = departure;
planes[i]->destination = destination;
planes[i]->date = date;
planes[i]->ticketNum = ticketNum;
planes[i]->ticketPrice = ticketPrice;
break;
}
}
}
void showPlanes() {
for (int i = 0; i < planes.size(); i++) {
cout << planes[i]->id << " " << planes[i]->departure << " " << planes[i]->destination << " " << planes[i]->date << " " << planes[i]->ticketNum << " " << planes[i]->ticketPrice << endl;
}
}
Plane* findPlane(string id) {
for (int i = 0; i < planes.size(); i++) {
if (planes[i]->id == id) {
return planes[i];
}
}
return NULL;
}
void saveToFile(string filename) {
ofstream fout(filename);
fout << name << " " << password << endl;
if (user == NULL) {
fout << "NULL" << endl;
} else {
fout << user->name << " " << user->age << " " << user->id << endl;
}
fout << planes.size() << endl;
for (int i = 0; i < planes.size(); i++) {
fout << planes[i]->id << " " << planes[i]->departure << " " << planes[i]->destination << " " << planes[i]->date << " " << planes[i]->ticketNum << " " << planes[i]->ticketPrice << endl;
}
fout.close();
}
void loadFromFile(string filename) {
ifstream fin(filename);
fin >> name >> password;
string userName;
fin >> userName;
if (userName == "NULL") {
user = NULL;
} else {
int age;
string id;
fin >> age >> id;
user = new User(userName, age, id);
}
int num;
fin >> num;
for (int i = 0; i < num; i++) {
string id, departure, destination, date;
int ticketNum, ticketPrice;
fin >> id >> departure >> destination >> date >> ticketNum >> ticketPrice;
planes.push_back(new Plane(id, departure, destination, date, ticketNum, ticketPrice));
}
fin.close();
}
private:
string name;
string password;
User* user;
vector<Plane*> planes;
};
int main() {
Manager manager("admin", "admin");
manager.loadFromFile("data.txt");
string command;
while (true) {
cout << "请输入指令:";
cin >> command;
if (command == "login") {
string name, password;
cout << "请输入用户名和密码:";
cin >> name >> password;
if (manager.login(name, password)) {
cout << "登录成功!" << endl;
} else {
cout << "用户名或密码错误!" << endl;
}
} else if (command == "addUser") {
string name, id;
int age;
cout << "请输入用户姓名、年龄、身份证号:";
cin >> name >> age >> id;
manager.addUser(new User(name, age, id));
cout << "添加用户成功!" << endl;
} else if (command == "addPlane") {
string id, departure, destination, date;
int ticketNum, ticketPrice;
cout << "请输入航班号、起点、终点、日期、票数、票价:";
cin >> id >> departure >> destination >> date >> ticketNum >> ticketPrice;
manager.addPlane(new Plane(id, departure, destination, date, ticketNum, ticketPrice));
cout << "添加航班成功!" << endl;
} else if (command == "deletePlane") {
string id;
cout << "请输入要删除的航班号:";
cin >> id;
manager.deletePlane(id);
cout << "删除航班成功!" << endl;
} else if (command == "modifyPlane") {
string id, departure, destination, date;
int ticketNum, ticketPrice;
cout << "请输入要修改的航班号、起点、终点、日期、票数、票价:";
cin >> id >> departure >> destination >> date >> ticketNum >> ticketPrice;
manager.modifyPlane(id, departure, destination, date, ticketNum, ticketPrice);
cout << "修改航班成功!" << endl;
} else if (command == "findPlane") {
string id;
cout << "请输入要查询的航班号:";
cin >> id;
Plane* plane = manager.findPlane(id);
if (plane == NULL) {
cout << "找不到航班!" << endl;
} else {
cout << "航班号:" << plane->id << " 起点:" << plane->departure << " 终点:" << plane->destination << " 日期:" << plane->date << " 票数:" << plane->ticketNum << " 票价:" << plane->ticketPrice << endl;
}
} else if (command == "showPlanes") {
manager.showPlanes();
} else if (command == "saveToFile") {
string filename;
cout << "请输入文件名:";
cin >> filename;
manager.saveToFile(filename);
cout << "保存成功!" << endl;
} else if (command == "loadFromFile") {
string filename;
cout << "请输入文件名:";
cin >> filename;
manager.loadFromFile(filename);
cout << "加载成功!" << endl;
} else if (command == "exit") {
break;
} else {
cout << "无效指令!" << endl;
}
}
manager.saveToFile("data.txt");
return 0;
}
```
其中,数据结构文件保存了管理员的用户名、密码、用户信息和航班信息。管理员的用户名和密码保存在第一行,如果没有用户信息,则在第二行写入“NULL”,否则在第二行写入用户的姓名、年龄和身份证号。航班数量保存在第三行,之后每一行写入一条航班的航班号、起点、终点、日期、票数和票价。
相关推荐







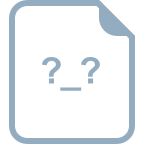
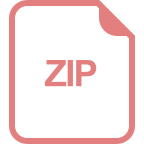
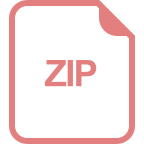
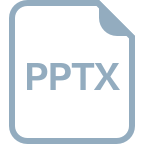