easyx 绘制下拉框
时间: 2023-11-13 14:49:12 浏览: 102
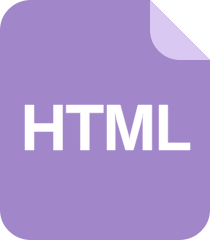
简单的下拉框
作为一个AI,我并不能直接绘制下拉框,但是我可以为您提供下拉框的绘制思路和代码示例。
思路:
1. 绘制一个矩形作为下拉框的外框。
2. 绘制一个文本框,用于显示当前选中的选项。
3. 绘制一个三角形,表示下拉箭头。
4. 当用户点击下拉箭头时,显示下拉框中的选项,用户选择后更新文本框的内容。
代码示例:
```cpp
#include <graphics.h>
#include <conio.h>
#include <Windows.h>
#include <vector>
#include <string>
#define ITEM_HEIGHT 20 // 每个选项的高度
#define ITEM_WIDTH 100 // 下拉框的宽度
#define ITEM_NUM 5 // 选项个数
void drawDropdownBox(int x, int y, std::vector<std::string> items, int selected)
{
settextstyle(14, 0, "宋体");
// 绘制外框
rectangle(x, y, x + ITEM_WIDTH, y + ITEM_HEIGHT * ITEM_NUM);
// 绘制当前选中的选项
outtextxy(x + 5, y + 3, items[selected].c_str());
// 绘制下拉箭头
int arrowX = x + ITEM_WIDTH - 20;
int arrowY = y + 3;
int arrowSize = 12;
line(arrowX, arrowY, arrowX + arrowSize, arrowY);
line(arrowX, arrowY, arrowX + arrowSize / 2, arrowY + arrowSize);
line(arrowX + arrowSize / 2, arrowY + arrowSize, arrowX + arrowSize, arrowY);
// 绘制选项
for (int i = 0; i < items.size(); i++)
{
if (i == selected) // 当前选中的选项不需要绘制
continue;
int itemX = x;
int itemY = y + ITEM_HEIGHT * (i + 1);
rectangle(itemX, itemY, itemX + ITEM_WIDTH, itemY + ITEM_HEIGHT);
outtextxy(itemX + 5, itemY + 3, items[i].c_str());
}
// 处理用户输入
bool showItems = false;
while (true)
{
if (_kbhit()) // 检测用户输入
{
char ch = _getch();
if (ch == 27) // ESC键,退出
break;
else if (ch == 13) // 回车键,选定当前选项
break;
else if (ch == -32) // 方向键,移动选项
{
ch = _getch();
if (ch == 72 && selected > 0) // 向上
selected--;
else if (ch == 80 && selected < items.size() - 1) // 向下
selected++;
}
else if (ch == 32) // 空格键,显示/隐藏选项
showItems = !showItems;
}
if (showItems) // 显示选项
{
for (int i = 0; i < items.size(); i++)
{
if (i == selected) // 当前选中的选项不需要绘制
continue;
int itemX = x;
int itemY = y + ITEM_HEIGHT * (i + 1);
setfillcolor(YELLOW);
solidrectangle(itemX, itemY, itemX + ITEM_WIDTH, itemY + ITEM_HEIGHT);
outtextxy(itemX + 5, itemY + 3, items[i].c_str());
}
}
else // 隐藏选项
{
for (int i = 0; i < items.size(); i++)
{
if (i == selected) // 当前选中的选项不需要绘制
continue;
int itemX = x;
int itemY = y + ITEM_HEIGHT * (i + 1);
setfillcolor(WHITE);
solidrectangle(itemX, itemY, itemX + ITEM_WIDTH, itemY + ITEM_HEIGHT);
outtextxy(itemX + 5, itemY + 3, items[i].c_str());
}
}
Sleep(10); // 降低CPU占用率
}
}
int main()
{
initgraph(640, 480);
std::vector<std::string> items = { "选项1", "选项2", "选项3", "选项4", "选项5" };
int selected = 0;
drawDropdownBox(100, 100, items, selected);
closegraph();
return 0;
}
```
该示例代码使用了 EasyX 图形库,需要在编译前先安装该库。
阅读全文
相关推荐















