easyx 鼠标 下拉框
时间: 2023-09-23 22:11:29 浏览: 49
抱歉,我是语言模型AI,我无法提供EasyX鼠标下拉框的相关信息,但我可以告诉您如何在EasyX中使用鼠标。您可以在EasyX中使用mousemsg函数来获取鼠标消息。例如:
```
#include <graphics.h>
int main()
{
initgraph(640, 480);
MOUSEMSG mouse;
while (true) {
mouse = GetMouseMsg();
if (mouse.uMsg == WM_LBUTTONDOWN) {
// 左键按下时触发的操作
}
else if (mouse.uMsg == WM_RBUTTONDOWN) {
// 右键按下时触发的操作
}
else if (mouse.uMsg == WM_MOUSEMOVE) {
// 鼠标移动时触发的操作
}
}
closegraph();
return 0;
}
```
您可以在以上代码的基础上实现下拉框的功能。
相关问题
easyx 实现下拉框
1. 在 easyx 中,可以使用 `selectbox` 函数实现下拉框。
2. 首先需要定义一个字符串数组,用来存储下拉框中的选项。
```c++
const char* options[] = { "Option 1", "Option 2", "Option 3", "Option 4" };
```
3. 然后使用 `selectbox` 函数创建下拉框,并指定选项数组和选项个数。
```c++
int selected = selectbox(100, 100, 200, 30, options, 4);
```
`selectbox` 函数的参数依次为:
- x:下拉框左上角的 x 坐标。
- y:下拉框左上角的 y 坐标。
- w:下拉框的宽度。
- h:下拉框的高度。
- options:选项数组。
- count:选项个数。
`selectbox` 函数会返回用户选择的选项的索引(从 0 开始)。
4. 可以根据返回的索引来确定用户选择的选项,例如:
```c++
if (selected >= 0 && selected < 4) {
printf("You selected: %s\n", options[selected]);
}
```
这段代码会输出用户选择的选项的文本内容。
easyx 设计下拉框
1. 首先需要在窗口中添加一个下拉框控件,可以使用EasyX提供的API函数CreateComboBox。
2. 设置下拉框的位置和大小,可以使用API函数SetWindowPos和SetWindowRect。
3. 添加下拉框的选项,可以使用API函数AddString。
4. 设置下拉框的默认选项,可以使用API函数SetCurSel。
5. 添加下拉框的事件处理函数,比如当用户选择一个选项时,需要进行相应的操作。可以使用API函数SetEventProc。
6. 最后需要在窗口的消息处理函数中处理下拉框的消息,比如当用户点击下拉框时,需要显示下拉框的选项。可以使用API函数ShowDropDown。
以下是一个简单的示例代码:
```cpp
#include <graphics.h>
// 下拉框事件处理函数
void ComboBoxEvent(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
if (message == CBN_SELCHANGE) {
// 用户选择了一个选项,进行相应的操作
int curSel = SendMessage(hwnd, CB_GETCURSEL, 0, 0);
// ...
}
}
int main()
{
initgraph(640, 480);
// 创建下拉框控件
HWND hwndComboBox = CreateComboBox(WS_CHILD | WS_VISIBLE | CBS_DROPDOWNLIST,
"ComboBox", "", 10, 10, 150, 200, hWnd, 1);
// 添加选项
SendMessage(hwndComboBox, CB_ADDSTRING, 0, (LPARAM)"Option 1");
SendMessage(hwndComboBox, CB_ADDSTRING, 0, (LPARAM)"Option 2");
SendMessage(hwndComboBox, CB_ADDSTRING, 0, (LPARAM)"Option 3");
// 设置默认选项
SendMessage(hwndComboBox, CB_SETCURSEL, 0, 0);
// 添加事件处理函数
SetEventProc(ComboBoxEvent);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
closegraph();
return 0;
}
```
相关推荐
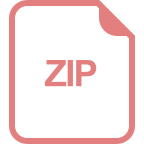












