如何给tableview的数据排序
时间: 2024-03-26 20:35:42 浏览: 61
在PyQt中,我们可以使用QTableView的setSortingEnabled()方法来使表格中的数据可以排序。具体来说,你可以在创建QTableView对象后,使用setSortingEnabled(True)方法启用排序功能,示例如下:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QStandardItem, QStandardItemModel
from PyQt5.QtWidgets import QApplication, QTableView
# 创建一个QStandardItemModel对象
model = QStandardItemModel()
# 添加数据到model中...
# 创建一个QTableView对象,并设置model
table_view = QTableView()
table_view.setModel(model)
# 启用排序功能
table_view.setSortingEnabled(True)
# 显示窗口
window = QApplication([])
table_view.show()
window.exec_()
```
在上面的示例代码中,我们使用了setSortingEnabled(True)方法启用了QTableView的排序功能。这样,当用户单击表头时,表格中的数据就会按照所选列的值进行排序。需要注意的是,如果数据模型中的数据发生了变化,排序可能会失效,因此你需要在修改数据后重新启用排序功能。
相关问题
tableview排序
在 iOS 中,可以使用 `NSSortDescriptor` 类来对 `UITableView` 中的数据进行排序。以下是一个示例,假设我们有一个包含 `Person` 对象的数组 `peopleArray`,每个 `Person` 对象都有一个 `name` 属性和一个 `age` 属性。
首先,我们需要创建一个 `NSSortDescriptor` 对象来指定我们要按照哪个属性进行排序:
```swift
let nameSortDescriptor = NSSortDescriptor(key: "name", ascending: true)
let ageSortDescriptor = NSSortDescriptor(key: "age", ascending: true)
```
上面的代码创建了两个 `NSSortDescriptor` 对象,一个按照 `name` 属性升序排序,另一个按照 `age` 属性升序排序。
接下来,我们需要将这些 `NSSortDescriptor` 对象传递给 `sortedArray(using:)` 方法来对数组进行排序。在这个例子中,我们将使用 `nameSortDescriptor` 对数组进行排序:
```swift
let sortedPeopleArray = (peopleArray as NSArray).sortedArray(using: [nameSortDescriptor]) as! [Person]
```
上面的代码将 `peopleArray` 数组按照 `name` 属性升序排序,结果存储在 `sortedPeopleArray` 数组中。如果你想按照多个属性排序,只需将 `NSSortDescriptor` 对象放入数组中即可:
```swift
let sortedPeopleArray = (peopleArray as NSArray).sortedArray(using: [nameSortDescriptor, ageSortDescriptor]) as! [Person]
```
上面的代码将 `peopleArray` 数组按照 `name` 属性升序排序,如果有相同的 `name` 属性,则按照 `age` 属性升序排序。
qttableview更新数据
在 Qt 中,要更新 QTableView 组件中的数据,可以通过以下步骤进行操作:
1. 获取对应的数据模型:QTableView 组件通常是基于 QAbstractTableModel 或其子类实现的。因此,首先需要获取到 QTableView 组件关联的数据模型对象。
```cpp
QAbstractTableModel* model = qobject_cast<QAbstractTableModel*>(tableView->model());
```
2. 更新数据:根据你的需求,更新数据模型中的数据。这可以通过修改模型中的数据项或重新加载数据来完成。
```cpp
// 修改单个数据项
model->setData(model->index(row, column), newValue, Qt::EditRole);
// 重新加载数据
model->layoutChanged();
```
如果你想一次性更新多个数据项,可以使用 `model->setData()` 方法来设置多个数据项的值,然后再调用 `model->submit()` 来提交更改。
3. 刷新视图:更新数据后,需要刷新 QTableView 组件的视图以显示更新后的数据。
```cpp
tableView->viewport()->update();
```
通过执行以上步骤,你就可以在 QTableView 组件中成功更新数据。记得根据实际情况进行相应的调整和错误处理。
阅读全文
相关推荐
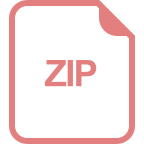
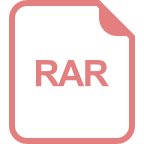
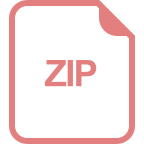
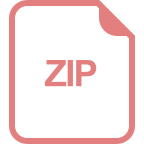
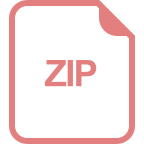
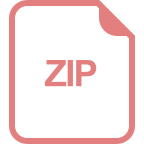
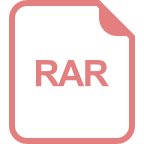
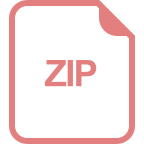
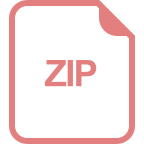
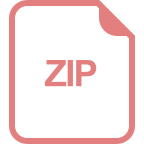



