phase coding 代码
时间: 2023-07-02 09:05:30 浏览: 112
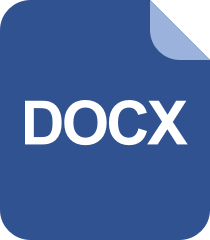
C#代码规范(微软内部资料).docx
Phase coding is a technique used in digital signal processing to modulate the phase of a signal in order to encode information. Here's an example of phase coding code in Python:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define the phase coding function
def phase_encode(info, freq, duration, sampling_rate):
time = np.linspace(0, duration, int(duration * sampling_rate), endpoint=False)
phase = np.zeros_like(time)
for i, bit in enumerate(info):
if bit == 1:
phase[i*sampling_rate//freq:(i+1)*sampling_rate//freq] = np.pi
signal = np.sin(2*np.pi*freq*time + phase)
return signal
# Define the parameters
info = [1, 0, 1, 1, 0, 0] # binary information to encode
freq = 10 # frequency of the carrier signal
duration = len(info) # duration of the signal in seconds
sampling_rate = 100 # sampling rate of the signal in Hz
# Generate the phase-coded signal
signal = phase_encode(info, freq, duration, sampling_rate)
# Plot the signal
plt.plot(signal)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.show()
```
In this code, we define a `phase_encode` function that takes in binary information to encode, the frequency of the carrier signal, the duration of the signal, and the sampling rate. The function generates a sinusoidal signal with a phase that is modulated to encode the binary information. We then define some parameters and call the `phase_encode` function to generate the signal. Finally, we plot the signal using `matplotlib.pyplot`.
阅读全文
相关推荐
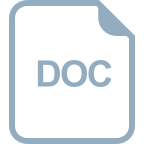
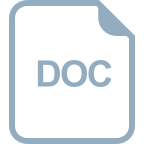
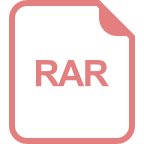
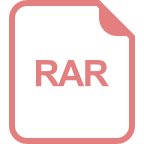
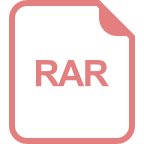
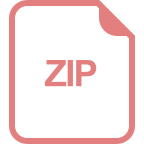
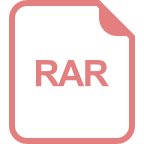
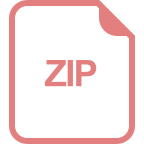
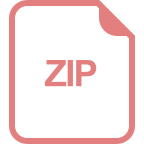
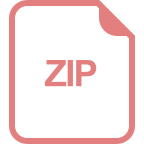
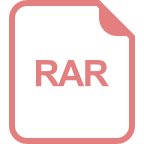
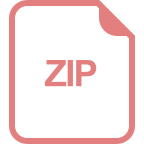
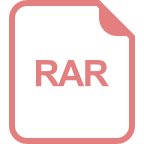
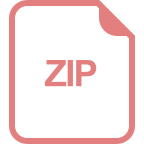
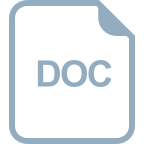
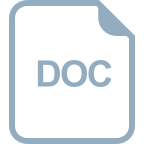

