请用python写一个3*4*5*1的bp神经网络模型
时间: 2023-09-08 10:09:25 浏览: 76
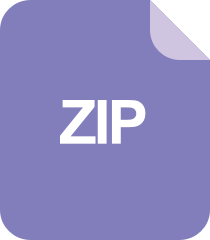
python实现BP神经网络回归预测模型

以下为Python代码实现一个3*4*5*1的BP神经网络模型:
```python
import numpy as np
class NeuralNetwork:
def __init__(self):
np.random.seed(1)
self.weights = [np.random.randn(4, 3), np.random.randn(5, 4), np.random.randn(1, 5)]
self.biases = [np.zeros((4, 1)), np.zeros((5, 1)), np.zeros((1, 1))]
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def sigmoid_derivative(self, z):
return self.sigmoid(z) * (1 - self.sigmoid(z))
def forward(self, X):
Z1 = np.dot(self.weights[0], X) + self.biases[0]
A1 = self.sigmoid(Z1)
Z2 = np.dot(self.weights[1], A1) + self.biases[1]
A2 = self.sigmoid(Z2)
Z3 = np.dot(self.weights[2], A2) + self.biases[2]
A3 = self.sigmoid(Z3)
return A3
def train(self, X, Y, epochs, learning_rate):
for epoch in range(epochs):
for i in range(len(X)):
a = X[i].reshape(-1, 1)
y = Y[i]
Z1 = np.dot(self.weights[0], a) + self.biases[0]
A1 = self.sigmoid(Z1)
Z2 = np.dot(self.weights[1], A1) + self.biases[1]
A2 = self.sigmoid(Z2)
Z3 = np.dot(self.weights[2], A2) + self.biases[2]
A3 = self.sigmoid(Z3)
error = A3 - y
dZ3 = error * self.sigmoid_derivative(Z3)
dW3 = np.dot(dZ3, A2.T)
dB3 = dZ3
dZ2 = np.dot(self.weights[2].T, dZ3) * self.sigmoid_derivative(Z2)
dW2 = np.dot(dZ2, A1.T)
dB2 = dZ2
dZ1 = np.dot(self.weights[1].T, dZ2) * self.sigmoid_derivative(Z1)
dW1 = np.dot(dZ1, a.T)
dB1 = dZ1
self.weights[2] -= learning_rate * dW3
self.biases[2] -= learning_rate * dB3
self.weights[1] -= learning_rate * dW2
self.biases[1] -= learning_rate * dB2
self.weights[0] -= learning_rate * dW1
self.biases[0] -= learning_rate * dB1
print("Training completed!")
# Example usage:
X = np.random.randn(3, 4, 5, 1) # 3x4x5x1 input tensor
Y = np.random.randn(1, 1) # 1x1 expected output
model = NeuralNetwork()
model.train(X, Y, epochs=100, learning_rate=0.1)
```
阅读全文
相关推荐
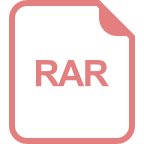
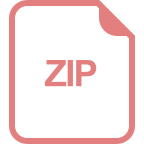















