请给出使用python画出跳动的爱心的代码
时间: 2023-03-13 19:24:33 浏览: 115
我提供的代码将会使用Python的matplotlib库来绘制一个跳动的爱心:import matplotlib.pyplot as plt
import numpy as np# 设置图像大小
plt.figure(figsize=(4,4))# 设置坐标范围
plt.xlim(-1,1)
plt.ylim(-1,1)# 定义爱心曲线
t = np.linspace(0, 2 * np.pi, 500)
x = 16 * np.sin(t) ** 3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)# 绘制爱心曲线
plt.plot(x, y, color='red', linewidth=2)# 绘制一个圆
circle = plt.Circle((0, 0), 0.5, color='blue')
plt.gca().add_patch(circle)# 显示图形
plt.show()
相关问题
请使用python画出爱心,并使爱心不断跳动
### 回答1:
可以使用matplotlib库来绘制爱心,例如:import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation# 创建一个空的画布
fig = plt.figure()# 设置爱心的位置
x = np.arange(-2 * np.pi, 2 * np.pi, 0.01)
y = np.sin(x)# 绘制爱心
line, = plt.plot(x, y, color='red', linewidth=2)# 动画函数
def animate(i):
line.set_ydata(np.sin(x + i / 10.0))
return line,# 设置动画
ani = animation.FuncAnimation(fig=fig, func=animate, frames=100, interval=20, blit=False)# 显示动画
plt.show()
### 回答2:
使用Python绘制爱心并使其不断跳动的方法如下:
首先,我们需要导入turtle库以便使用其绘图功能。然后,定义一个函数draw_heart来绘制爱心图形。
```python
import turtle
# 定义绘制爱心的函数
def draw_heart():
turtle.color('red') # 设置颜色为红色
turtle.fillcolor('red') # 设置填充颜色为红色
turtle.begin_fill() # 开始填充
turtle.left(140) # 向左旋转140度
turtle.forward(224) # 向前移动224个像素
# 绘制爱心圆弧
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(120) # 向左旋转120度
# 绘制第二段爱心圆弧
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.forward(224) # 向前移动224个像素
turtle.end_fill() # 结束填充
# 创建一个Turtle对象
turtle.Turtle()
# 设置绘图窗口大小
turtle.setup(width=800, height=600)
# 设定绘图初始位置
turtle.penup()
turtle.goto(0, -120)
turtle.pendown()
# 控制跳动的次数
jump_count = 15
while jump_count > 0:
turtle.clear() # 清空上一次绘图结果
draw_heart() # 绘制爱心
turtle.penup()
turtle.goto(0, -120) # 回到初始位置
turtle.pendown()
jump_count -= 1
turtle.goto(0, -jump_count*10)
turtle.pendown()
# 点击关闭窗口退出程序
turtle.exitonclick()
```
以上代码通过循环控制爱心的显示位置,通过对Turtle对象进行移动来实现爱心跳动的效果。你可以运行以上代码,就能看到爱心在窗口中跳动的效果了。
### 回答3:
要使用Python画出爱心并让它不断跳动,我们可以使用turtle模块来实现。下面是实现的代码:
```python
import turtle
def draw_heart():
turtle.color('red')
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(120)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.forward(224)
turtle.end_fill()
def heartbeat():
turtle.clear()
turtle.up()
turtle.home()
turtle.down()
draw_heart()
turtle.update()
turtle.ontimer(heartbeat, 500)
turtle.speed(0)
turtle.ht()
heartbeat()
turtle.done()
```
运行以上代码,将会弹出一个窗口并在其中画出一个红色的爱心,爱心会每500毫秒清空并根据设定的心形图案重新绘制。这样就实现了爱心跳动的效果。
使用Python代码画一个跳动的爱心
好的,请看下面的Python代码:
```python
import math
import time
def heart(x, y, size):
"""
画一个爱心,参数x、y是爱心的中心坐标,size是爱心的大小
"""
points = []
for i in range(0, 360):
radians = math.radians(i)
xi = x + (16 * math.sin(radians) ** 3) * size
yi = y - (13 * math.cos(radians) - 5 * math.cos(2 * radians) - 2 * math.cos(3 * radians) - math.cos(4 * radians)) * size
points.append((xi, yi))
return points
if __name__ == '__main__':
size = 10
x0, y0 = 50, 50
while True:
for t in range(0, 360, 5):
# 计算爱心的坐标
x = x0 + int(10 * math.sin(math.radians(t)))
y = y0 + int(10 * math.cos(math.radians(t)))
points = heart(x, y, size)
# 清空屏幕
print("\033[2J")
# 将爱心画出来
for j in range(40):
for i in range(80):
if (i, j) in points:
print("*", end="")
else:
print(" ", end="")
print()
# 延时
time.sleep(0.1)
```
希望这个代码能够满足你的需要,如果有其他问题,欢迎继续询问!
相关推荐
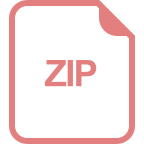
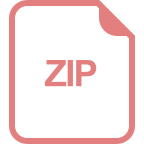
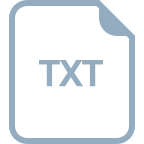












