public static void main(String[] args) { Scanner in = new Scanner(System.in); //先判断类 String task = "SystemFramework"; String dity = "GroupLeader"; String str = in.next(); int length = str.length(); 如何判断str输入的是回车
时间: 2023-12-18 21:02:24 浏览: 27
要判断用户输入的是否为回车,可以使用`Scanner`类的`nextLine()`方法来读取用户输入,并将其存储在一个字符串中。然后判断这个字符串是否为空字符串,如果是,则说明用户只输入了回车。
具体代码如下:
```
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String task = "SystemFramework";
String dity = "GroupLeader";
String str = in.nextLine(); // 使用 nextLine() 方法读取用户输入
int length = str.length();
if (length == 0) {
System.out.println("用户输入了回车");
} else {
System.out.println("用户输入的字符串为:" + str);
}
}
```
相关问题
import java.util.Scanner; /** * 定义InvalidInputException异常类,继承自Exception */ class InvalidInputException extends Exception { public InvalidInputException(String message) { super(message); } } public class Task { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("请输入年龄: "); int age = scanner.nextInt(); // TODO 如果输入的年龄小于0或者大于200,抛出InvalidInputException异常 System.out.println("您输入的年龄是: " + age); } } }
您好!对于您的问题,您可以在输入年龄的代码块中添加条件判断,如果年龄小于0或者大于200,就抛出InvalidInputException异常。修改后的代码如下所示:
```java
import java.util.Scanner;
/**
* 定义InvalidInputException异常类,继承自Exception
*/
class InvalidInputException extends Exception {
public InvalidInputException(String message) {
super(message);
}
}
public class Task {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请输入年龄: ");
int age = scanner.nextInt();
// 如果输入的年龄小于0或者大于200,抛出InvalidInputException异常
if (age < 0 || age > 200) {
try {
throw new InvalidInputException("年龄无效");
} catch (InvalidInputException e) {
System.out.println(e.getMessage());
continue;
}
}
System.out.println("您输入的年龄是: " + age);
}
}
}
```
这样,如果用户输入的年龄小于0或者大于200,程序会抛出InvalidInputException异常,并打印出异常的信息。然后程序会继续循环,等待用户重新输入年龄。
希望以上回答对您有所帮助!如果您有任何其他问题,请随时提问。
public class StudentDemo { public static void main(String[] args) { Scanner in = new Scanner(System.in); //判断类型并循环输入 String stuNo = in.next(); String name = in.next(); double english = in.nextDouble(); double math = in.nextDouble(); double Java = in.nextDouble(); String str = in.nextLine(); double testScore = 1.0; for(int i=0;i<3;i++){ //学生PT if(str.equals(" ")){//如果输入回车 Student s1 = new StudentPT(stuNo,name,english,math,Java,testScore); System.out.println(s1.toString()); } else if(str.equals("SystemFramework")){ Student s2 = new StudentPT(stuNo,name,english,math,Java,testScore); System.out.println(s2.toString() + " task:SystemFramework"); } else if(str.equals("GroupLeader")){ Student s3 = new StudentZZ(stuNo,name,english,math,Java,testScore); System.out.println(s3.toString() + " duty:GroupLeader"); } } 无输出
代码中有几个问题需要注意:
1. 在输入字符串 `str` 时,应该使用 `in.nextLine()` 而不是 `in.next()`,因为 `next()` 只会读取输入的下一个单词,若输入了回车键,则会被忽略掉。
2. 在循环中,每次都要重新读取 `str` 的值,否则会陷入死循环。
3. 循环的条件 `i<3` 是固定的,应该根据实际需求进行判断。
下面是修正后的代码:
```
public class StudentDemo {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
// 判断类型并循环输入
String stuNo, name, str;
double english, math, Java, testScore = 1.0;
while (in.hasNextLine()) {
stuNo = in.next();
name = in.next();
english = in.nextDouble();
math = in.nextDouble();
Java = in.nextDouble();
in.nextLine(); // 读取回车符
str = in.nextLine();
// 学生PT
if (str.equals("")) {
Student s1 = new StudentPT(stuNo, name, english, math, Java, testScore);
System.out.println(s1.toString());
} else if (str.equals("SystemFramework")) {
Student s2 = new StudentPT(stuNo, name, english, math, Java, testScore);
System.out.println(s2.toString() + " task:SystemFramework");
} else if (str.equals("GroupLeader")) {
Student s3 = new StudentZZ(stuNo, name, english, math, Java, testScore);
System.out.println(s3.toString() + " duty:GroupLeader");
} else {
break;
}
}
}
}
```
此代码可以一直输入直到用户停止输入,若输入回车则会跳出循环。
相关推荐
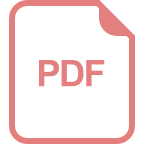
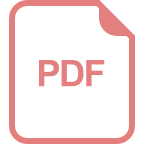
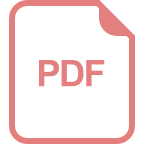








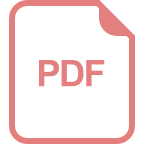
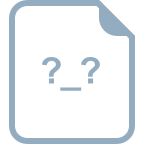
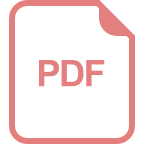
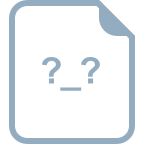
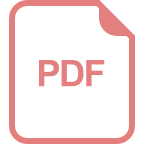