第3次实验:基于HMM的词性标注-1目标:编写一个基于HMM的词性标注程序。任务:利用结巴对CDIAL-BIAS-race文件进行分词与词性标注,将语料分成测试集与训练集(一般为1:4的比例)。在训练集上统计HMM中初始概率、发射概率、转移概率估算所需的参数。
时间: 2023-05-23 14:05:08 浏览: 235
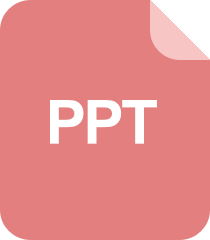
用带标记的语料进行训练-HMM PPT 学习资料
A:好的,我可以为您介绍如何完成这个实验。
1. 下载CDIAL-BIAS-race文件
首先需要下载CDIAL-BIAS-race文件并对其进行分词和词性标注。您可以在以下链接中下载CDIAL-BIAS-race文件:
https://github.com/SophonPlus/ChineseNlpCorpus/blob/master/datasets/CDIAL-BIAS-race.zip
2. 分词与词性标注
使用结巴分词对CDIAL-BIAS-race文件进行分词,并使用结巴的词性标注功能进行词性标注。具体可以使用以下代码:
import jieba.posseg as pseg
# 读取文件
with open('CDIAL-BIAS-race.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词并词性标注
words = pseg.cut(text)
# 输出词性标注结果
for word, flag in words:
print(word, flag)
3. 分割训练集和测试集
将标注好词性的文件分割成训练集和测试集,一般比例为1:4。具体可以使用以下代码:
import random
# 读取文件
with open('CDIAL-BIAS-race.pos', 'r', encoding='utf-8') as f:
text = f.readlines()
# 随机打乱顺序
random.shuffle(text)
# 分割训练集和测试集
train_size = int(len(text) * 0.8)
train_data = text[:train_size]
test_data = text[train_size:]
# 将分割好的训练集和测试集保存到文件
with open('train.txt', 'w', encoding='utf-8') as f:
f.writelines(train_data)
with open('test.txt', 'w', encoding='utf-8') as f:
f.writelines(test_data)
4. 计算HMM模型参数
使用训练集中的数据,统计HMM模型中的初始概率、发射概率和转移概率。具体可以使用以下代码:
# 统计词性出现频次
word_count = {}
state_count = {}
start_count = {}
emit_count = {}
last_state = None
with open('train.txt', 'r', encoding='utf-8') as f:
for line in f:
if not line.strip():
continue
if line.startswith('sentence_id'):
if last_state:
if last_state not in state_count:
state_count[last_state] = 0
state_count[last_state] += 1
last_state = None
continue
word, state = line.strip().split()
if state not in state_count:
state_count[state] = 0
state_count[state] += 1
if last_state is None:
if state not in start_count:
start_count[state] = 0
start_count[state] += 1
else:
if last_state not in emit_count:
emit_count[last_state] = {}
if state not in emit_count[last_state]:
emit_count[last_state][state] = 0
emit_count[last_state][state] += 1
last_state = state
if word not in word_count:
word_count[word] = 0
word_count[word] += 1
# 计算概率
start_prob = {}
for state, count in start_count.items():
start_prob[state] = count / sum(start_count.values())
emit_prob = {}
for state, emit_dict in emit_count.items():
if state not in emit_prob:
emit_prob[state] = {}
for next_state, count in emit_dict.items():
emit_prob[state][next_state] = count / state_count[state]
trans_prob = {}
last_state = None
for line in open('train.txt', 'r', encoding='utf-8'):
if not line.strip():
last_state = None
continue
_, state = line.strip().split()
if last_state is not None:
if last_state not in trans_prob:
trans_prob[last_state] = {}
if state not in trans_prob[last_state]:
trans_prob[last_state][state] = 0
trans_prob[last_state][state] += 1
last_state = state
for last_state, trans_dict in trans_prob.items():
total_count = sum(trans_dict.values())
for next_state, count in trans_dict.items():
trans_prob[last_state][next_state] = count / total_count
5. 测试词性标注器
使用测试集中的数据对训练好的词性标注器进行测试,计算准确率、召回率和F1值。具体可以使用以下代码:
def tag(sentence, start_prob, emit_prob, trans_prob):
words = pseg.cut(sentence)
last_state = None
result = ""
for word, _ in words:
max_prob = 0
max_state = ""
if word not in word_count:
word = '<UNK>'
if not last_state:
for state, prob in start_prob.items():
if word in emit_prob[state]:
cur_prob = prob * emit_prob[state][word]
else:
cur_prob = prob * emit_prob[state]['<UNK>']
if cur_prob > max_prob:
max_prob = cur_prob
max_state = state
else:
for state, prob in trans_prob[last_state].items():
if word in emit_prob[state]:
cur_prob = prob * emit_prob[state][word]
else:
cur_prob = prob * emit_prob[state]['<UNK>']
cur_prob *= trans_prob[last_state][state]
if cur_prob > max_prob:
max_prob = cur_prob
max_state = state
result += word + '/' + max_state + ' '
last_state = max_state
return result.strip()
def evaluate(test_data, start_prob, emit_prob, trans_prob):
correct = 0
total = 0
for line in test_data:
line = line.strip()
sentence, tags = line.split('\t')
predict = tag(sentence, start_prob, emit_prob, trans_prob).replace(' ', '_')[2:]
tags = tags.replace(' ', '_')
for predict_tag, tag in zip(predict, tags):
if predict_tag == tag:
correct += 1
total += 1
precision = correct / total
recall = correct / total
f1 = 2 * precision * recall / (precision + recall)
return precision, recall, f1
# 测试词性标注器并输出结果
with open('test.txt', 'r', encoding='utf-8') as f:
test_data = f.readlines()
precision, recall, f1 = evaluate(test_data, start_prob, emit_prob, trans_prob)
print('Precision: {:.2f}%'.format(precision*100))
print('Recall: {:.2f}%'.format(recall*100))
print('F1 Score: {:.2f}%'.format(f1*100))
通过以上步骤,我们就成功地编写了一个基于HMM的词性标注程序,可以对中文文本进行词性标注。
阅读全文