用c++写一个ras加解密算法
时间: 2024-02-25 11:57:07 浏览: 164
以下是使用Crypto++库实现的RAS加解密算法的C++代码示例:
```cpp
#include <iostream>
#include <string>
#include <cryptopp/rsa.h>
#include <cryptopp/osrng.h>
#include <cryptopp/pssr.h>
#include <cryptopp/base64.h>
using namespace std;
using namespace CryptoPP;
int main()
{
AutoSeededRandomPool rng; // 随机数生成器
// 生成RSA密钥对
RSA::PrivateKey privateKey;
RSA::PublicKey publicKey;
privateKey.GenerateRandomWithKeySize(rng, 2048); // 私钥长度为2048位
publicKey.AssignFrom(privateKey);
// 加密
string message = "Hello, world!";
string ciphertext;
RSAES_OAEP_SHA_Encryptor encryptor(publicKey);
StringSource(message, true, new PK_EncryptorFilter(rng, encryptor, new StringSink(ciphertext)));
// 输出密文
string encoded;
StringSource(ciphertext, true, new Base64Encoder(new StringSink(encoded)));
cout << "Encoded ciphertext: " << encoded << endl;
// 解密
string decrypted;
RSAES_OAEP_SHA_Decryptor decryptor(privateKey);
StringSource(encoded, true, new Base64Decoder(new PK_DecryptorFilter(rng, decryptor, new StringSink(decrypted))));
// 输出明文
cout << "Decrypted message: " << decrypted << endl;
return 0;
}
```
以上代码使用Crypto++库实现了RSA密钥对的生成、加密和解密操作,并使用Base64编码输出密文。在实际使用中,需要根据具体需求进行修改和扩展。
阅读全文
相关推荐
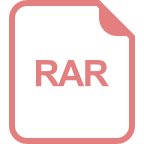
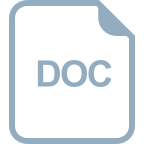
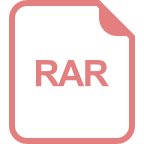
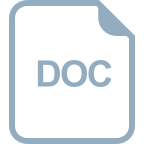
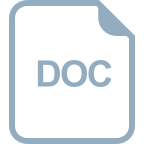
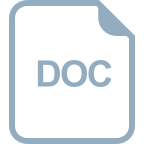


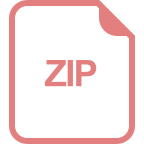
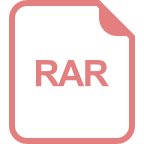
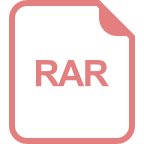
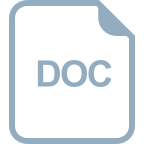
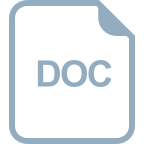
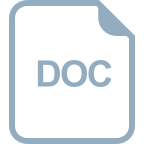
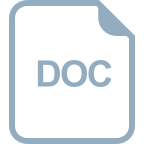