unity 在shader中设置字体颜色
时间: 2024-04-28 21:19:08 浏览: 302
在Unity中设置字体颜色的方法是使用Shader,具体步骤如下:
1. 创建一个新的Shader,可以在Unity编辑器中使用“Create > Shader > Standard Surface Shader”选项创建。
2. 在Shader中添加一个属性来控制字体颜色,可以使用“Properties”块中的“Color”类型。
3. 在Shader中的“SubShader”块中添加一个“Pass”块,用来渲染字体。
4. 在“Pass”块中使用“CGPROGRAM”和“ENDCG”块来定义Shader代码。
5. 在Shader代码中使用“UNITY_INSTANCING_BUFFER_START”和“UNITY_INSTANCING_BUFFER_END”宏来实现GPU实例化,从而提高渲染性能。
6. 在Shader代码中使用“UNITY_ACCESS_INSTANCED_PROP”宏来访问实例化属性,例如字体颜色。
7. 在Shader代码中使用“UNITY_DEFINE_INSTANCED_PROP”宏来定义实例化属性,例如字体颜色。
8. 在Shader代码中使用“UNITY_TRANSFER_INSTANCE_ID”宏来实现GPU实例化,从而提高渲染性能。
9. 在Shader代码中使用“UNITY_VERTEX_INPUT_INSTANCE_ID”宏来实现GPU实例化,从而提高渲染性能。
10. 最后,在Unity中使用该Shader渲染字体时,将字体颜色属性设置为所需的颜色即可。
以上步骤可以实现在Unity中设置字体颜色的功能。
相关问题
unity 2d流光shader
Unity 2D流光shader是一种可用于渲染2D图形的着色器效果,其使用了特殊的技术来模拟流光效果。该效果能够通过改变颜色和亮度来让图像看起来像是有移动的光线在画面上流动。
流光shader是使用Unity ShaderLab编写的,其中包含了一系列的OpenGL语言和Unity自定义的着色器语言,并可以自由调整各个参数。例如,流光的强度,颜色,方向等等,都可以通过调整shader中的参数来实现。
这种效果在游戏设计中具有非常广泛的应用。流光shader可以用来增强游戏场景的气氛,比如可以用来制作神秘的夜晚效果、地下城效果等等。此外,在游戏菜单和UI设计上,也可以使用流光shader来为菜单字体、按钮等元素添加动态效果,从而使游戏更加生动。
总之,Unity 2D流光shader 是一种非常有趣的2D图形渲染效果,它可以给游戏增加流动感,同时也为游戏的视觉效果和用户体验带来了更多的乐趣。
Unity 中使用自定义颜色给字体和图片换颜色
在Unity中,可以使用自定义颜色来给字体和图片换颜色。下面是具体的步骤:
1. 创建一个新的Material,将Shader设置为UI/Default。
2. 在Inspector窗口中,找到Color属性,点击右侧的颜色选择器,选择你想要的颜色。
3. 将这个新的Material赋给你想要改变颜色的字体或图片的Material。
4. 如果你想动态地改变颜色,可以在脚本中使用以下代码:
```
// 获取字体或图片的Renderer组件
Renderer renderer = GetComponent<Renderer>();
// 获取之前创建的Material
Material material = renderer.material;
// 设置颜色
material.color = new Color(1f, 0f, 0f, 1f);
```
这里的new Color(1f, 0f, 0f, 1f)表示设置颜色为红色,alpha值为1。你可以根据需要自己设置颜色。
阅读全文
相关推荐
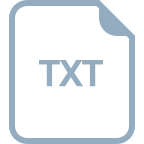
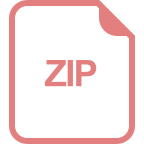
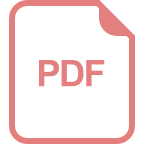
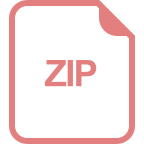
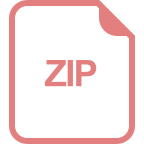
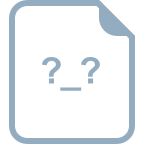
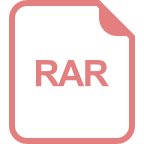


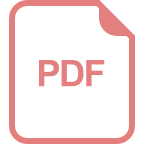
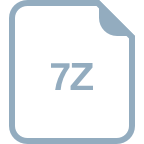
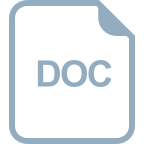
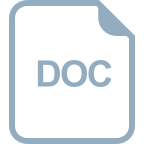

