哈夫曼树,第一行输入一个数n,表示叶结点的个数。 需要用这些叶结点生成哈夫曼树,根据哈夫曼树的概念,这些结点有权值,即weight,题目需要输出哈夫曼树的带权路径长度(WPL)。
时间: 2023-07-08 07:55:16 浏览: 112
好的,我来给出一个实现。首先,我们需要定义一个节点类来表示哈夫曼树中的节点,包含权值和左右子节点。代码如下:
```python
class HuffmanNode:
def __init__(self, weight, left=None, right=None):
self.weight = weight
self.left = left
self.right = right
```
接着,我们需要构建哈夫曼树。我们可以通过一个优先队列来实现,每次取出权值最小的两个节点,合并成一个新节点,并将其放回优先队列中,直到只剩下一个节点。代码如下:
```python
import heapq
def build_huffman_tree(weights):
"""
Build a Huffman tree from a list of weights.
"""
nodes = [HuffmanNode(weight) for weight in weights]
heapq.heapify(nodes)
while len(nodes) > 1:
left = heapq.heappop(nodes)
right = heapq.heappop(nodes)
parent = HuffmanNode(left.weight + right.weight, left, right)
heapq.heappush(nodes, parent)
return nodes[0]
```
最后,我们需要计算哈夫曼树的带权路径长度。遍历树的所有叶子节点,将每个叶子节点的权值乘以其到根节点的距离,然后将所有结果相加即可。代码如下:
```python
def huffman_wpl(root, depth=0):
"""
Calculate the WPL of a Huffman tree.
"""
if not root:
return 0
if not root.left and not root.right:
return root.weight * depth
return huffman_wpl(root.left, depth+1) + huffman_wpl(root.right, depth+1)
```
完整代码如下:
```python
import heapq
class HuffmanNode:
def __init__(self, weight, left=None, right=None):
self.weight = weight
self.left = left
self.right = right
def build_huffman_tree(weights):
"""
Build a Huffman tree from a list of weights.
"""
nodes = [HuffmanNode(weight) for weight in weights]
heapq.heapify(nodes)
while len(nodes) > 1:
left = heapq.heappop(nodes)
right = heapq.heappop(nodes)
parent = HuffmanNode(left.weight + right.weight, left, right)
heapq.heappush(nodes, parent)
return nodes[0]
def huffman_wpl(root, depth=0):
"""
Calculate the WPL of a Huffman tree.
"""
if not root:
return 0
if not root.left and not root.right:
return root.weight * depth
return huffman_wpl(root.left, depth+1) + huffman_wpl(root.right, depth+1)
n = int(input())
weights = list(map(int, input().split()))
root = build_huffman_tree(weights)
wpl = huffman_wpl(root)
print(wpl)
```
这样,我们就可以输入叶子节点个数和权值,输出哈夫曼树的带权路径长度了。
阅读全文
相关推荐
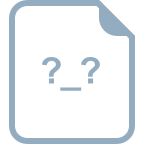
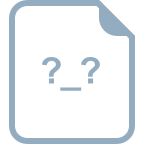














