怎么复制文件夹并创建C语言
时间: 2024-05-07 11:20:10 浏览: 74
要在C语言中复制文件夹,需要使用系统调用来执行操作。以下是一个简单的示例程序,它使用系统调用来复制文件夹:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <sys/types.h>
int copy(char *source, char *destination) {
DIR *dir;
struct dirent *entry;
char src_path[1024], dst_path[1024];
struct stat statbuf;
if ((dir = opendir(source)) == NULL) {
printf("无法打开源文件夹: %s\n", source);
return -1;
}
mkdir(destination, S_IRWXU | S_IRWXG | S_IROTH | S_IXOTH);
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
sprintf(src_path, "%s/%s", source, entry->d_name);
sprintf(dst_path, "%s/%s", destination, entry->d_name);
if (stat(src_path, &statbuf) == -1) {
printf("无法获取文件信息: %s\n", src_path);
continue;
}
if (S_ISDIR(statbuf.st_mode)) {
copy(src_path, dst_path);
} else {
FILE *fsrc, *fdst;
char buffer[1024];
int n;
if ((fsrc = fopen(src_path, "rb")) == NULL) {
printf("无法打开源文件: %s\n", src_path);
continue;
}
if ((fdst = fopen(dst_path, "wb")) == NULL) {
printf("无法创建目标文件: %s\n", dst_path);
fclose(fsrc);
continue;
}
while ((n = fread(buffer, sizeof(char), sizeof(buffer), fsrc)) > 0) {
if (fwrite(buffer, sizeof(char), n, fdst) != n) {
printf("写入文件时出错: %s\n", dst_path);
fclose(fsrc);
fclose(fdst);
remove(dst_path);
continue;
}
}
fclose(fsrc);
fclose(fdst);
}
}
closedir(dir);
return 0;
}
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("使用方法: %s 源文件夹 目标文件夹\n", argv[0]);
return 0;
}
if (copy(argv[1], argv[2]) == 0) {
printf("复制文件夹成功!\n");
} else {
printf("复制文件夹失败!\n");
}
return 0;
}
```
该程序首先打开源文件夹,创建目标文件夹,然后遍历源文件夹中的每个文件和子文件夹。对于每个子文件夹,程序递归地调用自己,以便复制子文件夹中的内容。对于每个文件,程序打开源文件和目标文件,并使用 fread 和 fwrite 函数复制文件内容。如果复制过程中出现错误,程序会打印出错误信息并继续复制其他文件。最后,程序关闭所有打开的文件和文件夹,并返回复制结果。
阅读全文
相关推荐
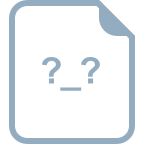
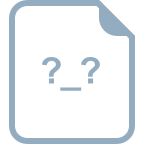
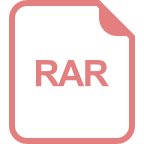
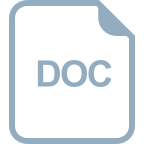










