C语言写图书管理系统,要有登录注册存储借书信息功能
时间: 2024-05-04 13:17:45 浏览: 154
好的,下面是一个简单的C语言图书管理系统的代码示例,包括登录、注册、存储和借书功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_USERS 100 // 最大用户数
#define MAX_BOOKS 1000 // 最大图书数
#define MAX_BORROWS 1000 // 最大借阅数
// 用户结构体
typedef struct {
char username[20]; // 用户名
char password[20]; // 密码
} User;
// 图书结构体
typedef struct {
char title[50]; // 书名
char author[20]; // 作者
char publisher[20]; // 出版社
int year; // 出版年份
int id; // 图书编号
int borrowed; // 是否借出
} Book;
// 借阅结构体
typedef struct {
char username[20]; // 用户名
int book_id; // 图书编号
} Borrow;
int user_count = 0; // 用户数
User users[MAX_USERS]; // 用户数组
int book_count = 0; // 图书数
Book books[MAX_BOOKS]; // 图书数组
int borrow_count = 0; // 借阅数
Borrow borrows[MAX_BORROWS]; // 借阅数组
// 登录函数
int login() {
char username[20], password[20];
printf("请输入用户名:");
scanf("%s", username);
printf("请输入密码:");
scanf("%s", password);
for (int i = 0; i < user_count; i++) {
if (strcmp(users[i].username, username) == 0 && strcmp(users[i].password, password) == 0) {
printf("登录成功!\n");
return i;
}
}
printf("用户名或密码错误!\n");
return -1;
}
// 注册函数
void register_user() {
if (user_count >= MAX_USERS) {
printf("用户数已达到上限!\n");
return;
}
char username[20], password[20];
printf("请输入用户名:");
scanf("%s", username);
printf("请输入密码:");
scanf("%s", password);
for (int i = 0; i < user_count; i++) {
if (strcmp(users[i].username, username) == 0) {
printf("该用户名已被注册!\n");
return;
}
}
strcpy(users[user_count].username, username);
strcpy(users[user_count].password, password);
user_count++;
printf("注册成功!\n");
}
// 添加图书函数
void add_book() {
if (book_count >= MAX_BOOKS) {
printf("图书数已达到上限!\n");
return;
}
char title[50], author[20], publisher[20];
int year;
printf("请输入书名:");
scanf("%s", title);
printf("请输入作者:");
scanf("%s", author);
printf("请输入出版社:");
scanf("%s", publisher);
printf("请输入出版年份:");
scanf("%d", &year);
books[book_count].id = book_count + 1;
strcpy(books[book_count].title, title);
strcpy(books[book_count].author, author);
strcpy(books[book_count].publisher, publisher);
books[book_count].year = year;
books[book_count].borrowed = 0;
book_count++;
printf("添加成功!\n");
}
// 显示所有图书函数
void show_books() {
printf("图书编号\t书名\t作者\t出版社\t出版年份\t是否借出\n");
for (int i = 0; i < book_count; i++) {
printf("%d\t%s\t%s\t%s\t%d\t%s\n", books[i].id, books[i].title, books[i].author, books[i].publisher, books[i].year, books[i].borrowed ? "是" : "否");
}
}
// 借书函数
void borrow_book(int user_index) {
if (borrow_count >= MAX_BORROWS) {
printf("借阅数已达到上限!\n");
return;
}
int book_id;
printf("请输入要借的图书编号:");
scanf("%d", &book_id);
if (book_id <= 0 || book_id > book_count) {
printf("该图书不存在!\n");
return;
}
if (books[book_id - 1].borrowed) {
printf("该图书已被借出!\n");
return;
}
strcpy(borrows[borrow_count].username, users[user_index].username);
borrows[borrow_count].book_id = book_id;
books[book_id - 1].borrowed = 1;
borrow_count++;
printf("借阅成功!\n");
}
// 还书函数
void return_book(int user_index) {
int book_id;
printf("请输入要还的图书编号:");
scanf("%d", &book_id);
if (book_id <= 0 || book_id > book_count) {
printf("该图书不存在!\n");
return;
}
for (int i = 0; i < borrow_count; i++) {
if (strcmp(borrows[i].username, users[user_index].username) == 0 && borrows[i].book_id == book_id) {
books[book_id - 1].borrowed = 0;
for (int j = i; j < borrow_count - 1; j++) {
borrows[j] = borrows[j + 1];
}
borrow_count--;
printf("还书成功!\n");
return;
}
}
printf("您没有借阅该图书!\n");
}
int main() {
int choice, user_index = -1;
while (1) {
printf("欢迎使用图书管理系统!\n");
printf("1.登录 2.注册 3.退出\n");
scanf("%d", &choice);
if (choice == 1) {
user_index = login();
if (user_index == -1) {
continue;
}
} else if (choice == 2) {
register_user();
continue;
} else if (choice == 3) {
break;
} else {
printf("无效的选项!\n");
continue;
}
while (1) {
printf("1.添加图书 2.显示所有图书 3.借书 4.还书 5.退出\n");
scanf("%d", &choice);
if (choice == 1) {
add_book();
} else if (choice == 2) {
show_books();
} else if (choice == 3) {
borrow_book(user_index);
} else if (choice == 4) {
return_book(user_index);
} else if (choice == 5) {
break;
} else {
printf("无效的选项!\n");
}
}
}
return 0;
}
```
需要注意的是,上述代码仅为示例,实际应用中还需要考虑更多的情况,比如输入的数据是否合法、数据存储方式等等。此外,代码中的数据存储是在内存中,如果需要实现永久存储,需要使用文件等外部存储方式。
阅读全文
相关推荐
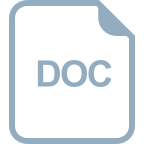
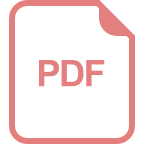
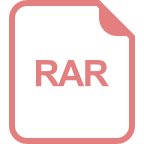
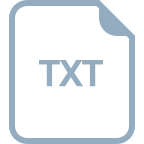
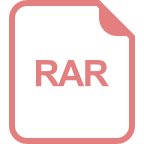
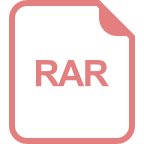
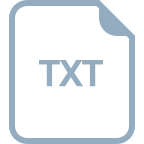
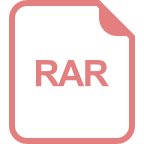
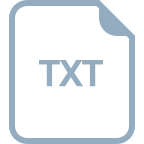
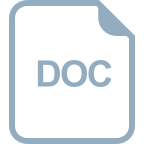
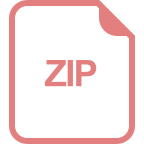
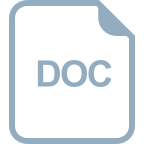





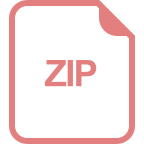