#include<iostream> using namespace std; class Point{ private: int x; int y; public: Point& operator++() { ++x; ++y; return*this; } Point& operator+(Point &p1) { Point temp; temp.x=p1.x+x; temp.y=p1.y+y; return temp; } Point(int x = 0,int y =0) { this->x=x; this->y=y; } friend Point operator--(Point&,int); friend Point operator-(Point,Point); friend ostream& operator<<(ostream &stm,Point &p); }; Point operator--(Point &p2,int) { p2.x++; p2.y++; return p2; } Point operator-(Point &p1,Point &p2) { Point temp; temp.x=p1.x-p2.x; temp.y=p1.y-p2.y; return temp; } ostream& operator<<(ostream &stm,Point &p) { stm<<"("<<p.x<<","<<p.y<<")"<<endl; } int main() { Point p1, p2, p; cin >> p1 >> p2; p = ++p1; cout << "p=++p1后,p的坐标为:" << p << ",p1坐标为:" << p1 << endl; p = p2--; cout << "p=p2--后,p的坐标为:" << p << ",p2坐标为:" << p2 << endl; p = p1 + p2; cout << "p=p1+p2后,p的坐标为:" << p << endl; p = p1 - p2; cout << "p=p1-p2后,p的坐标为:" << p << endl; return 0; }找出错误
时间: 2024-03-06 10:48:14 浏览: 158
这段代码存在一些错误,包括:
1. 在运算符重载函数 operator+ 中,返回的 Point 对象使用了局部变量 temp,函数执行完毕后,temp 对象将被销毁,因此返回的是一个无效的对象。应该使用 new 在堆上创建对象,或者直接返回一个引用。
2. 在运算符重载函数 operator-- 中,后缀自减操作应该返回原始值,而不是自减后的值。
3. 在运算符重载函数 operator<< 中,应该返回输出流对象 stm,否则无法进行链式输出。
以下是修改后的代码:
```c++
#include<iostream>
using namespace std;
class Point{
private:
int x;
int y;
public:
Point& operator++() {
++x;
++y;
return *this;
}
const Point operator+(const Point& p1) const {
return Point(x + p1.x, y + p1.y);
}
Point operator--(int) {
Point temp = *this;
x--;
y--;
return temp;
}
const Point operator-(const Point& p) const {
return Point(x - p.x, y - p.y);
}
Point(int x = 0, int y = 0) {
this->x = x;
this->y = y;
}
friend ostream& operator<<(ostream& stm, const Point& p);
};
ostream& operator<<(ostream& stm, const Point& p) {
stm << "(" << p.x << "," << p.y << ")" << endl;
return stm;
}
int main() {
Point p1, p2, p;
cin >> p1 >> p2;
p = ++p1;
cout << "p=++p1后,p的坐标为:" << p << ",p1坐标为:" << p1 << endl;
p = p2--;
cout << "p=p2--后,p的坐标为:" << p << ",p2坐标为:" << p2 << endl;
p = p1 + p2;
cout << "p=p1+p2后,p的坐标为:" << p << endl;
p = p1 - p2;
cout << "p=p1-p2后,p的坐标为:" << p << endl;
return 0;
}
```
阅读全文
相关推荐
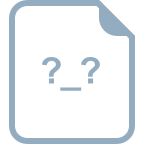
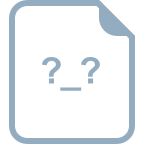










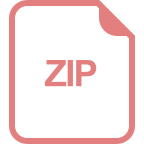
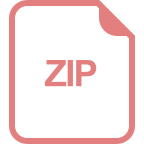