现有一个Person对象的列表如下: List<Person> people = new ArrayList<>(); people.add( new Person(25, "Alice Smith", LocalDate.of(1998, 5, 20))); people.add( new Person(30, "Bob Johnson", LocalDate.of1993, 10, 10))); people.add( new Person(30, "Charie Brown", LocalDate.of(1988, 3, 15))); people.add( new Person(40, "David Lee", LocalDate.of(1983, 7, 1)); people. .add(new Person(45, "Emily Chen", LocalDate.of(1978,12, 25))); age: 整数类型 dateOfBirth: 日期类型 fullName: 文本类型 使用java从people索引中查询年龄在30到40岁之间的文档,并按照年龄降序排序,打印出每个文档的ID和源
时间: 2024-03-17 14:40:59 浏览: 54
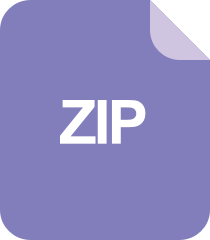
People:假设访问一组人员及其生日,编写一个方法,返回当前日历周生日的人员列表。 包括人名、生日所在的星期几以及他们的年龄
假设Person类包含id属性,可以按照以下方式查询并排序:
```java
List<Person> result = people.stream()
.filter(p -> p.getAge() >= 30 && p.getAge() <= 40)
.sorted(Comparator.comparingInt(Person::getAge).reversed())
.collect(Collectors.toList());
for (Person p : result) {
System.out.println("ID: " + p.getId() + ", Source: " + p);
}
```
注意,这里使用了Java 8的Stream API来过滤和排序列表。首先使用`filter`方法过滤出年龄在30到40岁之间的人,然后使用`sorted`方法按照年龄降序排序。最后使用`collect`方法将结果收集到一个新的列表中,并遍历打印出每个文档的ID和源。
阅读全文
相关推荐
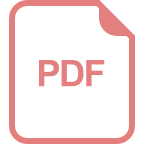
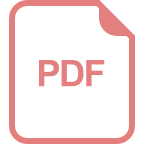

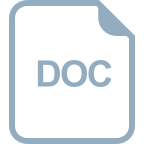
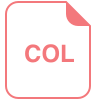













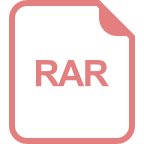