黄金矿工 C++ 多文件
时间: 2023-08-12 19:12:20 浏览: 41
黄金矿工是一个比较复杂的游戏,需要使用多个文件进行代码组织和管理。以下是一种可能的C++多文件实现方式:
1. main.cpp:主函数入口,负责初始化游戏界面和开始游戏。
```c++
#include "Game.h"
int main()
{
Game game;
game.init();
game.start();
return 0;
}
```
2. Game.h 和 Game.cpp:游戏类,负责游戏的逻辑处理和界面展示。
Game.h:
```c++
#ifndef GAME_H
#define GAME_H
#include "GoldMine.h"
#include "Player.h"
#include "UI.h"
class Game
{
public:
Game();
~Game();
void init();
void start();
private:
GoldMine* goldMine_;
Player* player_;
UI* ui_;
};
#endif
```
Game.cpp:
```c++
#include "Game.h"
Game::Game()
{
goldMine_ = new GoldMine();
player_ = new Player();
ui_ = new UI();
}
Game::~Game()
{
delete goldMine_;
delete player_;
delete ui_;
}
void Game::init()
{
goldMine_->init();
player_->init();
ui_->init();
}
void Game::start()
{
while (true)
{
goldMine_->update();
player_->update();
ui_->update();
if (goldMine_->isGameOver())
{
break;
}
}
}
```
3. GoldMine.h 和 GoldMine.cpp:金矿类,负责金矿的生成和更新。
GoldMine.h:
```c++
#ifndef GOLD_MINE_H
#define GOLD_MINE_H
#include "Gold.h"
class GoldMine
{
public:
GoldMine();
~GoldMine();
void init();
void update();
bool isGameOver();
private:
int width_;
int height_;
Gold*** golds_;
bool isGameOver_;
};
#endif
```
GoldMine.cpp:
```c++
#include "GoldMine.h"
GoldMine::GoldMine()
{
golds_ = nullptr;
}
GoldMine::~GoldMine()
{
if (golds_ != nullptr)
{
for (int i = 0; i < height_; i++)
{
for (int j = 0; j < width_; j++)
{
delete golds_[i][j];
}
delete[] golds_[i];
}
delete[] golds_;
}
}
void GoldMine::init()
{
width_ = 10;
height_ = 10;
golds_ = new Gold**[height_];
for (int i = 0; i < height_; i++)
{
golds_[i] = new Gold*[width_];
for (int j = 0; j < width_; j++)
{
golds_[i][j] = new Gold();
}
}
isGameOver_ = false;
}
void GoldMine::update()
{
// 更新金矿状态
// ...
}
bool GoldMine::isGameOver()
{
return isGameOver_;
}
```
4. Player.h 和 Player.cpp:玩家类,负责玩家的行动和得分。
Player.h:
```c++
#ifndef PLAYER_H
#define PLAYER_H
#include "Gold.h"
class Player
{
public:
Player();
~Player();
void init();
void update();
int getScore();
private:
int score_;
};
#endif
```
Player.cpp:
```c++
#include "Player.h"
Player::Player()
{
score_ = 0;
}
Player::~Player()
{
}
void Player::init()
{
score_ = 0;
}
void Player::update()
{
// 玩家行动逻辑
// ...
}
int Player::getScore()
{
return score_;
}
```
5. UI.h 和 UI.cpp:用户界面类,负责游戏画面的显示和交互。
UI.h:
```c++
#ifndef UI_H
#define UI_H
class UI
{
public:
UI();
~UI();
void init();
void update();
};
#endif
```
UI.cpp:
```c++
#include "UI.h"
UI::UI()
{
}
UI::~UI()
{
}
void UI::init()
{
// 初始化游戏界面
// ...
}
void UI::update()
{
// 更新游戏画面
// ...
}
```
6. Gold.h 和 Gold.cpp:金块类,表示一个金块的状态和价值。
Gold.h:
```c++
#ifndef GOLD_H
#define GOLD_H
class Gold
{
public:
Gold();
~Gold();
bool isAvailable();
int getValue();
private:
bool isAvailable_;
int value_;
};
#endif
```
Gold.cpp:
```c++
#include "Gold.h"
Gold::Gold()
{
isAvailable_ = true;
value_ = 10;
}
Gold::~Gold()
{
}
bool Gold::isAvailable()
{
return isAvailable_;
}
int Gold::getValue()
{
return value_;
}
```
以上就是一个简单的黄金矿工游戏的多文件实现方式。需要注意的是,每个类都需要有对应的头文件和源文件,并且头文件需要使用 #ifndef 和 #define 进行保护,以避免重复包含。
相关推荐









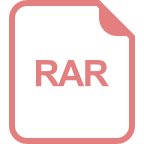