c++中,编写函数addStr,该函数的参数s指向一个仅由加法运算符和正整数组成的合法的表达式,函数计算表达式的值并返回。
时间: 2024-03-15 19:47:18 浏览: 23
可以使用栈来实现这个功能。具体步骤如下:
1. 遍历表达式字符串,遇到数字直接入栈,遇到加号则将下一个数字入栈,并将栈顶两个数字相加,将结果重新入栈。
2. 最后栈中只剩下一个数,即为表达式的结果。
下面是函数的实现代码:
```c++
int addStr(char* s) {
stack<int> nums;
int num = 0;
char op = '+';
while (*s) {
if (isdigit(*s)) {
num = num * 10 + (*s - '0');
}
if (!isdigit(*s) && (*s != ' ') || *(s + 1) == '\0') {
if (op == '+') {
nums.push(num);
}
else if (op == '-') {
nums.push(-num);
}
else if (op == '*') {
int n = nums.top();
nums.pop();
nums.push(n * num);
}
else if (op == '/') {
int n = nums.top();
nums.pop();
nums.push(n / num);
}
op = *s;
num = 0;
}
s++;
}
int res = 0;
while (!nums.empty()) {
res += nums.top();
nums.pop();
}
return res;
}
```
此函数可以处理加减乘除四则运算,对于其他运算符,可以根据需要进行扩展。
相关推荐
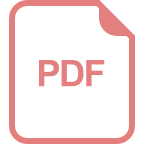
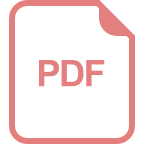
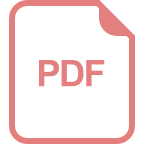














