编写一个贪吃蛇游戏的python代码
时间: 2024-12-13 21:24:19 浏览: 5
编写一个简单的贪吃蛇游戏Python代码需要一些基本的游戏元素,如屏幕、蛇的身体、食物、得分等。下面是一个基础版本的代码框架:
```python
import curses
import random
# 初始化窗口
stdscr = curses.initscr()
curses.curs_set(0) # 隐藏光标
# 设置窗口大小
win_height, win_width = stdscr.getmaxyx()
# 蛇的位置和方向
snake = [[5, 5], [4, 5], [3, 5]]
direction = 'down'
# 食物位置
food_x, food_y = random.randint(0, win_width - 1), random.randint(0, win_height - 1)
def move_snake():
new_head = snake[-1]
if direction == 'up':
new_head[0] -= 1
elif direction == 'down':
new_head[0] += 1
elif direction == 'left':
new_head[1] -= 1
else:
new_head[1] += 1
snake.insert(0, new_head)
if snake[0] == [food_x, food_y]:
score += 1
eat_food(food_x, food_y)
else:
tail = snake.pop()
stdscr.addch(tail[0], tail[1], ' ')
def eat_food(x, y):
global food_x, food_y
food_x, food_y = random.randint(0, win_width - 1), random.randint(0, win_height - 1)
while True:
for event in stdscr.getch(): # 检查用户输入
if event == ord('w') and direction != 'down':
direction = 'up'
elif event == ord('s') and direction != 'up':
direction = 'down'
elif event == ord('a') and direction != 'right':
direction = 'left'
elif event == ord('d') and direction != 'left':
direction = 'right'
move_snake()
stdscr.clear()
for body_part in snake:
stdscr.addch(body_part[0], body_part[1], '*')
stdscr.addstr(0, 0, f"Score: {score}")
stdscr.addch(food_x, food_y, '@')
stdscr.refresh()
curses.endwin() # 关闭窗口
```
这个代码只是一个非常简化的版本,实际游戏中还需要处理边界检查、碰撞检测以及玩家退出游戏等情况。如果你对游戏开发感兴趣,建议学习更专业的库如pygame或Kivy。
阅读全文
相关推荐
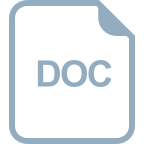
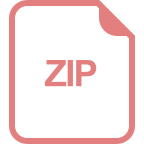
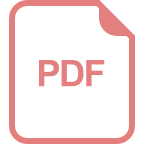









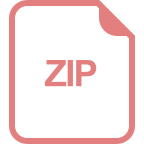
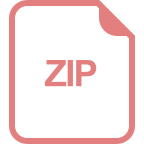
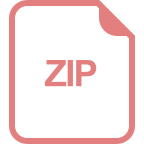
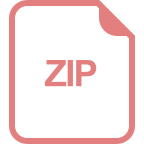
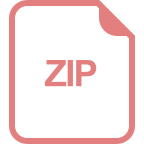