ConvergenceWarning: Maximum number of iteration reached before convergence. Consider increasing max_iter to improve the fit.
时间: 2023-06-18 10:03:00 浏览: 98
这个警告是说你的模型在迭代过程中没有收敛到一个稳定的状态,达到了最大迭代次数而停止了训练。这可能意味着你的模型过于复杂,或者你的数据集不够大,或者你的学习率设置不合适。你可以尝试增加最大迭代次数,或者减小模型的复杂度,或者调整学习率来解决这个问题。另外,你也可以检查一下你的数据集是否存在异常值或者缺失值等问题。
相关问题
if iteration == self.second_step_iter_num - 1: reg_noise_std = 0 elif iteration < 1000: reg_noise_std = (1 / 1000.) * (iteration // 100) else: reg_noise_std = 1 / 1000.
这段代码看起来是一个训练过程中的正则化(regularization)设置。根据iteration的不同阶段,使用不同的reg_noise_std值来对模型的权重进行加噪处理,以减少过拟合的情况。其中self.second_step_iter_num是训练过程中第二阶段的迭代次数,当iteration达到这个值时,就不再加噪了。如果iteration小于1000,则reg_noise_std随着iteration的增加而逐渐增加;否则reg_noise_std保持不变。具体来说,当iteration小于100时,每过100个iteration就将reg_noise_std增加1/1000;当iteration大于等于1000时,reg_noise_std就保持为1/1000。
完善以下代码:def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]: """ The em algorithm for aligning two point clouds based on affine transformation :param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points :param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points :param num_iter: the number of EM iterations :return: ys_new: the aligned points: ys_new = ys @ affine + translation responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M), whose elements indicating the correspondence between the points """ # TODO: implement the EM algorithm of GMM below for point cloud alignment return
Here is the implementation of the EM algorithm for point cloud alignment:
```python
from typing import Tuple
import numpy as np
def em_for_alignment(xs: np.ndarray, ys: np.ndarray, num_iter: int = 10) -> Tuple[np.ndarray, np.ndarray]:
"""
The em algorithm for aligning two point clouds based on affine transformation
:param xs: a set of points with size (N, D), N is the number of samples, D is the dimension of points
:param ys: a set of points with size (M, D), M is the number of samples, D is the dimension of points
:param num_iter: the number of EM iterations
:return:
ys_new: the aligned points: ys_new = ys @ affine + translation
responsibility: the responsibility matrix P=[p(y_m | x_n)] with size (N, M),
whose elements indicating the correspondence between the points
"""
# initialize the affine matrix and translation vector
affine = np.eye(xs.shape[1])
translation = np.zeros(xs.shape[1])
# initialize the responsibility matrix
responsibility = np.zeros((xs.shape[0], ys.shape[0]))
for i in range(num_iter):
# E-step: compute the responsibility matrix
for n in range(xs.shape[0]):
for m in range(ys.shape[0]):
responsibility[n, m] = 1 / (2 * np.pi) ** (xs.shape[1] / 2) * np.exp(
-0.5 * np.linalg.norm(xs[n] - ys[m] @ affine - translation) ** 2)
responsibility /= np.sum(responsibility, axis=1, keepdims=True)
# M-step: update the affine matrix and translation vector
xs_weighted = responsibility.T @ xs
ys_weighted = responsibility.T @ ys
affine, _, _, _ = np.linalg.lstsq(xs_weighted, ys_weighted, rcond=None)
translation = np.mean(ys, axis=0) - np.mean(xs @ affine, axis=0)
# compute the aligned points
ys_new = ys @ affine + translation
return ys_new, responsibility
```
The EM algorithm is used to estimate the affine matrix and translation vector that aligns the two point clouds. In each iteration, the algorithm computes the responsibility matrix that defines the correspondence between the points in the two clouds, and then updates the affine matrix and translation vector based on the weighted least squares solution. Finally, the algorithm computes the aligned points by applying the affine transformation to the original points and adding the translation vector.
相关推荐
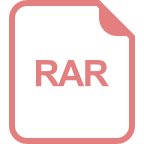
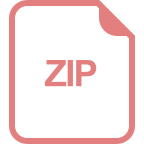













