java如何实现对Excel文件数据分组求和
时间: 2023-11-27 21:52:20 浏览: 34
Java可以使用Apache POI库来操作Excel文件,包括读取、写入、修改等操作,可以实现对Excel文件数据分组求和。
首先需要导入Apache POI库,然后打开Excel文件并获取要操作的Sheet,接着遍历每行数据,对需要分组的列进行判断,将相同的值分为一组,并将每组的数据进行求和,最后将结果写入到新的Excel文件中。
以下是示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelGroupSum {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream file = new FileInputStream(new File("data.xlsx"));
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0);
// 分组列的索引
int groupColumnIndex = 0;
// 存储每组的数据总和
Map<String, Double> groupSumMap = new HashMap<String, Double>();
// 遍历每行数据
for (Row row : sheet) {
// 跳过表头
if (row.getRowNum() == 0) {
continue;
}
// 获取分组列的值
Cell groupCell = row.getCell(groupColumnIndex);
String groupValue = groupCell.getStringCellValue();
// 获取需要求和的列的值
Cell sumCell = row.getCell(1);
double sumValue = sumCell.getNumericCellValue();
// 计算每组数据的总和
if (groupSumMap.containsKey(groupValue)) {
double oldSumValue = groupSumMap.get(groupValue);
groupSumMap.put(groupValue, oldSumValue + sumValue);
} else {
groupSumMap.put(groupValue, sumValue);
}
}
// 写入新的Excel文件
Workbook newWorkbook = new XSSFWorkbook();
Sheet newSheet = newWorkbook.createSheet("result");
int rowIndex = 0;
Row headerRow = newSheet.createRow(rowIndex++);
headerRow.createCell(0).setCellValue("分组列");
headerRow.createCell(1).setCellValue("总和");
for (Map.Entry<String, Double> entry : groupSumMap.entrySet()) {
Row dataRow = newSheet.createRow(rowIndex++);
dataRow.createCell(0).setCellValue(entry.getKey());
dataRow.createCell(1).setCellValue(entry.getValue());
}
FileOutputStream outputStream = new FileOutputStream("result.xlsx");
newWorkbook.write(outputStream);
// 关闭文件流
file.close();
newWorkbook.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
其中,`data.xlsx`是要操作的Excel文件,包含两列数据,第一列是需要分组的列,第二列是需要求和的列;`result.xlsx`是生成的新的Excel文件,包含两列数据,第一列是分组列的值,第二列是对应组的数据总和。可以根据实际需求修改分组列的索引、需要求和的列的索引以及文件名等参数。
相关推荐
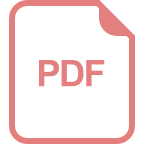
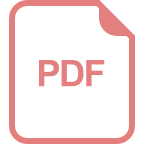













