C++语言代码实现二叉树的各种遍历算法的调试分析
时间: 2023-06-19 09:08:32 浏览: 94
首先,我们需要实现二叉树的数据结构。可以定义一个结构体来表示二叉树节点,包含节点值和左右子树指针。代码如下:
```c++
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
```
接下来,我们可以实现二叉树的各种遍历算法,包括前序遍历、中序遍历、后序遍历和层序遍历。这里我们以递归方式实现。代码如下:
```c++
// 前序遍历
void preOrder(TreeNode* root) {
if (root == nullptr) return;
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历
void inOrder(TreeNode* root) {
if (root == nullptr) return;
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
// 后序遍历
void postOrder(TreeNode* root) {
if (root == nullptr) return;
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
// 层序遍历
void levelOrder(TreeNode* root) {
if (root == nullptr) return;
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* cur = q.front();
q.pop();
cout << cur->val << " ";
if (cur->left != nullptr) q.push(cur->left);
if (cur->right != nullptr) q.push(cur->right);
}
}
```
接下来可以为这些函数编写测试代码,例如:
```c++
int main() {
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
root->right->left = new TreeNode(6);
root->right->right = new TreeNode(7);
cout << "Preorder: ";
preOrder(root);
cout << endl;
cout << "Inorder: ";
inOrder(root);
cout << endl;
cout << "Postorder: ";
postOrder(root);
cout << endl;
cout << "Level order: ";
levelOrder(root);
cout << endl;
return 0;
}
```
运行该程序,可以得到以下输出:
```
Preorder: 1 2 4 5 3 6 7
Inorder: 4 2 5 1 6 3 7
Postorder: 4 5 2 6 7 3 1
Level order: 1 2 3 4 5 6 7
```
如果需要调试二叉树的遍历算法,可以在遍历函数中添加打印语句,例如:
```c++
void preOrder(TreeNode* root) {
if (root == nullptr) return;
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
cout << "Finished processing node " << root->val << endl;
}
```
这样就可以在遍历二叉树的过程中打印出节点处理的顺序,方便调试。
阅读全文
相关推荐
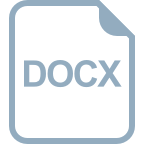
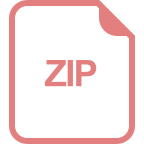
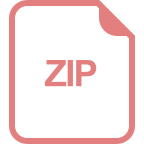










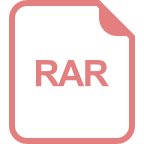
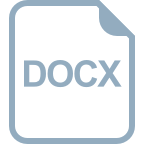
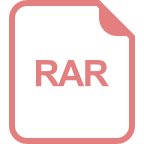
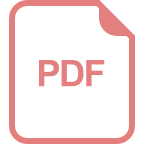
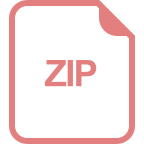
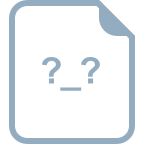