使用vue3+ts封装一个多选框函数
时间: 2024-01-24 21:04:04 浏览: 92
下面是一个使用 Vue3 和 TypeScript 封装的多选框函数示例:
```typescript
import { defineComponent, PropType } from 'vue'
interface Option {
label: string
value: string | number
}
export default defineComponent({
name: 'MultiSelect',
props: {
options: {
type: Array as PropType<Option[]>,
required: true
},
value: {
type: Array as PropType<(string | number)[]>,
default: () => []
}
},
emits: ['update:value'],
setup(props, { emit }) {
const isSelected = (value: string | number) => {
return props.value.includes(value)
}
const toggleOption = (value: string | number) => {
const idx = props.value.indexOf(value)
if (idx === -1) {
emit('update:value', [...props.value, value])
} else {
const newValue = [...props.value]
newValue.splice(idx, 1)
emit('update:value', newValue)
}
}
return {
isSelected,
toggleOption
}
},
template: `
<div>
<label v-for="option in options" :key="option.value">
<input type="checkbox" :value="option.value" v-model="isSelected(option.value)" @change="toggleOption(option.value)">
{{ option.label }}
</label>
</div>
`
})
```
使用时可以传入一个选项数组和一个值数组,选项数组包含多个选项对象,每个选项对象包含 `label` 和 `value` 两个属性,值数组包含已选的选项的值。组件会根据选项数组和值数组自动渲染多个复选框,并根据值数组设置复选框的选中状态。当用户勾选或取消勾选某个复选框时,组件会触发 `update:value` 事件并传递更新后的值数组。通过监听该事件,可以在父组件中更新值数组并实现多选框的功能。
阅读全文
相关推荐
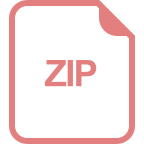
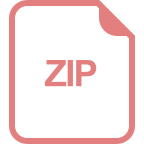




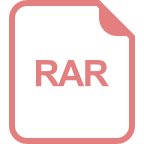











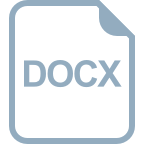