提取resnet网络任意层输出的代码怎么写
时间: 2023-09-11 16:05:12 浏览: 199
### 回答1:
可以使用PyTorch中的torch.utils.model_zoo模块,该模块可以轻松提取resnet网络中的任意层输出。示例代码如下:model = torch.utils.model_zoo.load_url('https://download.pytorch.org/models/resnet50-19c8e357.pth')
layer_output = model.layer[i] # 提取第i层输出
### 回答2:
要提取ResNet网络任意层输出,我们可以使用PyTorch提供的预训练模型和相应的函数来实现。下面是一个详细的代码示例:
首先,我们需要导入必要的库和模块:
```python
import torch
import torchvision.models as models
```
然后,我们可以选择一个预训练的ResNet模型,比如ResNet-50:
```python
resnet = models.resnet50(pretrained=True)
```
接下来,我们可以定义一个函数来提取任意层的输出。这个函数需要输入ResNet模型、输入数据和目标层的名称或索引:
```python
def get_layer_output(model, input, layer_name):
layer_output = None
def hook(module, input, output):
nonlocal layer_output
layer_output = output
# 获取目标层
target_layer = model._modules[layer_name]
# 注册钩子函数
handle = target_layer.register_forward_hook(hook)
# 前向传播
model(input)
# 删除钩子函数
handle.remove()
return layer_output
```
在这个函数中,我们首先定义了一个`hook`函数,该函数将被注册到目标层上,以获取该层的输出。然后,我们使用`register_forward_hook`方法将这个`hook`函数注册到目标层上。接着,我们进行一次前向传播以触发钩子函数并获取目标层的输出。最后,我们删除钩子函数并返回目标层的输出。
例如,如果我们想提取ResNet的第4个卷积层的输出,可以调用如下代码:
```python
input_data = torch.randn(1, 3, 224, 224) # 输入数据
layer_output = get_layer_output(resnet, input_data, 'layer4')
```
这样,我们就获得了ResNet第4个卷积层的输出`layer_output`,可以在接下来的处理中使用它。
希望这个回答能对你有所帮助!
### 回答3:
提取resnet网络任意层输出的代码的写法如下:
1. 首先,导入所需的库和模块:
```python
import torchvision.models as models
import torch
```
2. 加载预训练的ResNet模型(例如,ResNet50):
```python
resnet = models.resnet50(pretrained=True)
```
3. 设置模型为评估模式:
```python
resnet.eval()
```
4. 选择所需的层:
```python
layer_num = 4 # 层的编号,从0开始计算
selected_layer = resnet.layer[layer_num]
```
5. 构建一个前向传播的函数,该函数将会接受输入并获取指定层的输出:
```python
def get_selected_layer_output(x):
for index, layer in enumerate(selected_layer):
x = layer(x)
if index == layer_num:
return x
```
6. 输入一张图像或图像批量进行前向传播,然后获取指定层的输出:
```python
input_image = torch.randn(1, 3, 224, 224) # 输入的图像大小为(1, 3, 224, 224),可以根据实际情况修改
output = get_selected_layer_output(input_image)
```
上述代码将提取ResNet网络中第4个层的输出。如果需要提取不同的层,只需更改 `layer_num` 的值即可。
阅读全文
相关推荐
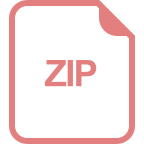
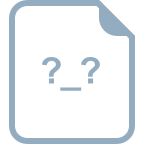
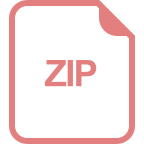
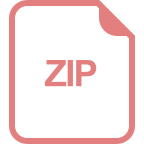
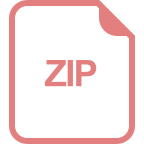
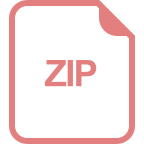
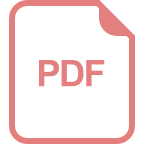
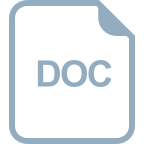
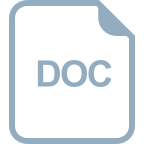
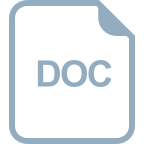
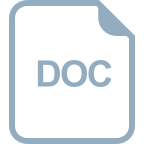
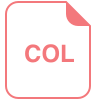
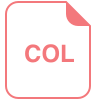
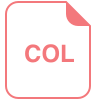
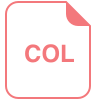
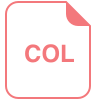
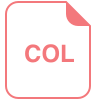
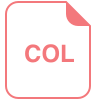
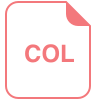