用C语言实现一个函数功能 输入:一个json文件, 要求:在这个json文件中增加一个参数“sig”,参数的值sign需要根据json文件内容计算得出。输出新的json文件。 参数的计算方法: 第一步: 将json文件所有非空参数拼接成一个stringA,拼接规则: 按照参数名ASCII码从小到大排序(字典序),使用URL键值对的格式(即key1=value1&key2=value2…)拼接成字符串 第二步:对stringA使用key进行HmacSHA256算法签名,得到sign
时间: 2024-02-01 11:14:06 浏览: 38
以下是一个使用C语言实现的函数,可以实现上述要求:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <openssl/hmac.h>
#include "cJSON.h"
char* calculate_sign(char* json_file, char* key) {
cJSON* root = cJSON_Parse(json_file);
if (!root) {
printf("Error parsing json file!\n");
return NULL;
}
int size = cJSON_GetArraySize(root);
char** keys = (char**) malloc(size * sizeof(char*));
char** values = (char**) malloc(size * sizeof(char*));
int i = 0;
cJSON* child = root->child;
while (child) {
char* key = child->string;
char* value = cJSON_PrintUnformatted(child);
keys[i] = key;
values[i] = value;
child = child->next;
i++;
}
// Sort keys in ascending order (using bubble sort)
for (i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (strcmp(keys[j], keys[j+1]) > 0) {
char* temp = keys[j];
keys[j] = keys[j+1];
keys[j+1] = temp;
temp = values[j];
values[j] = values[j+1];
values[j+1] = temp;
}
}
}
// Concatenate keys and values into a string
char* stringA = (char*) malloc(4096);
stringA[0] = '\0';
for (i = 0; i < size; i++) {
strcat(stringA, keys[i]);
strcat(stringA, "=");
strcat(stringA, values[i]);
if (i < size - 1) {
strcat(stringA, "&");
}
}
// Calculate HMAC-SHA256 of stringA using key
unsigned int len = strlen(key);
unsigned char* hmac = HMAC(EVP_sha256(), key, len, (unsigned char*)stringA, strlen(stringA), NULL, NULL);
// Convert HMAC to hexadecimal string
char* sign = (char*) malloc(65);
for (i = 0; i < 32; i++) {
sprintf(&sign[i*2], "%02x", hmac[i]);
}
sign[64] = '\0';
// Add "sig" parameter to the JSON object
cJSON_AddStringToObject(root, "sig", sign);
// Convert the JSON object back into a string
char* new_json_file = cJSON_PrintUnformatted(root);
// Free memory
for (i = 0; i < size; i++) {
free(keys[i]);
free(values[i]);
}
free(keys);
free(values);
free(stringA);
free(sign);
cJSON_Delete(root);
return new_json_file;
}
```
使用示例:
```c
int main() {
char* json_file = "{\"foo\": 123, \"bar\": \"hello\"}";
char* key = "my_secret_key";
char* new_json_file = calculate_sign(json_file, key);
printf("%s\n", new_json_file);
free(new_json_file);
return 0;
}
```
注意:需要在编译时链接 OpenSSL 库。例如,在 Linux 上可以使用以下命令编译:
```
gcc -o main main.c -lcrypto
```
相关推荐
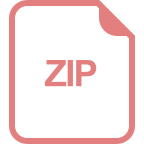
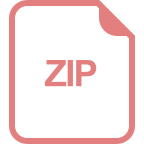














