怎么pytorch输出图片的shape
时间: 2024-05-12 22:16:40 浏览: 121
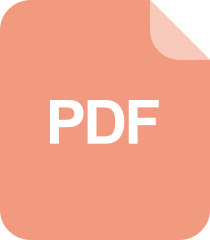
pytorch 实现将自己的图片数据处理成可以训练的图片类型
你可以通过pytorch中的torchvision模块中的transforms函数来将图片转换成tensor格式,然后使用torchvision.utils模块中的save_image函数将tensor保存成图片格式。在这个过程中可以使用tensor的shape属性来获取输出图片的shape。例如:
```python
import torch
import torchvision.transforms as transforms
from torchvision.utils import save_image
img_path = "my_image.png"
img_transform = transforms.Compose([
transforms.Resize((256, 256)),
transforms.ToTensor()
])
img_tensor = img_transform(Image.open(img_path))
print(img_tensor.shape) # 输出图片的shape
save_image(img_tensor, "output_image.png")
```
这段代码将图片打印出来的shape为三维的tensor,其中第一维代表了图片的通道数,第二和第三维分别代表了图片的高和宽。
阅读全文
相关推荐
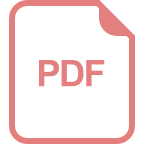
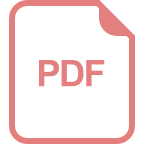


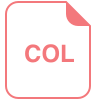
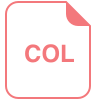
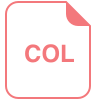
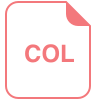









