public List<Student> QueryByName(string name) => students.Where((t) => t.Name.Contains(name)).ToList();
时间: 2024-05-25 10:19:13 浏览: 54
This method receives a string parameter "name" and returns a List of Student objects that match the search criteria. The search criteria is that the Name property of the Student object must contain the given "name" parameter.
The LINQ expression used to filter the students is:
students.Where((t) => t.Name.Contains(name))
This expression uses the Where() method to filter the students based on the given condition. The condition is specified using a lambda expression. The lambda expression takes a Student object "t" as input and checks if its Name property contains the given "name" parameter.
The filtered results are returned as a List using the ToList() method.
相关问题
public NormalMilitiaman queryByName(String name) { LambdaQueryWrapper<NormalMilitiaman> queryWrapper = new LambdaQueryWrapper<>(); queryWrapper.eq(NormalMilitiaman::getName, name); List<NormalMilitiaman> normalMilitiamanList = baseMapper.selectList(queryWrapper); if (normalMilitiamanList.size() == 1) { return normalMilitiamanList.get(0); } return null; }
这是一个Java代码段,它定义了一个查询方法queryByName,该方法通过名字(name)查询NormalMilitiaman对象。它使用了LambdaQueryWrapper对象来构建查询条件,使用eq方法来指定查询条件为name等于输入的name参数。然后使用baseMapper.selectList方法来查询数据库,将查询结果存储在一个List对象中,如果查询结果只有一个,则返回该对象,否则返回null。
```c++ #include <iostream> #include <string> #include <vector> using namespace std; struct Person { int id; string name; int age; char gender; }; vector<Person> persons = { {1, "Tom", 20, 'M'}, {2, "Lucy", 25, 'F'}, {3, "Bob", 30, 'M'}, }; printPerson(Person p) { cout << p.id << "\t" << p.name << "\t" << p.age << "\t" << p.gender << endl; } void queryByName(string name) { bool found = false; for (auto& p : persons) { if (p.name == name) { printPerson(p); found = true; } } if (!found) { cout << "No person with that name." << endl; } } int main() { cout << "ID\tName\tAge\tGender" << endl; for (auto& p : persons) { printPerson(p); } string name; cout << "Enter a name to query: "; cin >> name; queryByName(name); return 0; }这段代码有问题么
是的,这段代码有两个问题。
第一个问题是,在printPerson函数的定义中缺少返回类型。应该在函数定义前加上返回类型,例如void。
第二个问题是,在printPerson函数的调用中缺少参数。应该在函数名后加上括号并传入一个Person类型的参数,例如printPerson(p)。
以下是修正后的代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Person {
int id;
string name;
int age;
char gender;
};
vector<Person> persons = {
{1, "Tom", 20, 'M'},
{2, "Lucy", 25, 'F'},
{3, "Bob", 30, 'M'},
};
void printPerson(Person p) {
cout << p.id << "\t" << p.name << "\t" << p.age << "\t" << p.gender << endl;
}
void queryByName(string name) {
bool found = false;
for (auto& p : persons) {
if (p.name == name) {
printPerson(p);
found = true;
}
}
if (!found) {
cout << "No person with that name." << endl;
}
}
int main() {
cout << "ID\tName\tAge\tGender" << endl;
for (auto& p : persons) {
printPerson(p);
}
string name;
cout << "Enter a name to query: ";
cin >> name;
queryByName(name);
return 0;
}
```
阅读全文
相关推荐
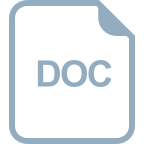
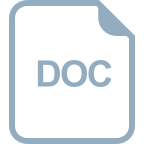



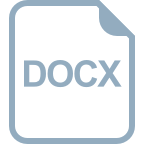
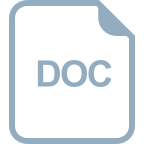
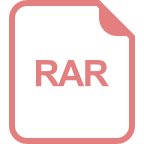









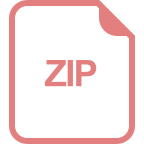