void queryByName() { char name[MAX_NAME_LENGTH]; printf("请输入姓名:"); scanf("%s", name); int found = 0; int i = 0; for (i; i < contactCount; i++) { if (strcmp(contacts[i].name, name) == 0) { printf("姓名:%s 工作单位:%s 电话号码:%s 电子邮件地址:%s\n", contacts[i].name, contacts[i].unit, contacts[i].phone, contacts[i].email); found = 1; } } if (!found) { printf("未找到该联系人。\n"); } }
时间: 2024-02-05 14:02:24 浏览: 49
这段代码是一个联系人管理程序中的查询联系人信息的函数。该函数会要求用户输入一个姓名,然后遍历联系人列表,查找姓名匹配的联系人,并输出其工作单位、电话号码和电子邮件地址。如果没有找到匹配的联系人,函数会输出一条提示信息。
需要注意的是,该函数中使用了字符串比较函数 `strcmp()` 来判断两个字符串是否相等,该函数在比较字符串时会返回一个整数,如果两个字符串相等,返回值为0。因此在判断字符串相等时,需要使用 `strcmp()` 返回值是否为0 来进行判断。
相关问题
```c++ #include <iostream> #include <string> #include <vector> using namespace std; struct Person { int id; string name; int age; char gender; }; vector<Person> persons = { {1, "Tom", 20, 'M'}, {2, "Lucy", 25, 'F'}, {3, "Bob", 30, 'M'}, }; printPerson(Person p) { cout << p.id << "\t" << p.name << "\t" << p.age << "\t" << p.gender << endl; } void queryByName(string name) { bool found = false; for (auto& p : persons) { if (p.name == name) { printPerson(p); found = true; } } if (!found) { cout << "No person with that name." << endl; } } int main() { cout << "ID\tName\tAge\tGender" << endl; for (auto& p : persons) { printPerson(p); } string name; cout << "Enter a name to query: "; cin >> name; queryByName(name); return 0; }这段代码有问题么
是的,这段代码有两个问题。
第一个问题是,在printPerson函数的定义中缺少返回类型。应该在函数定义前加上返回类型,例如void。
第二个问题是,在printPerson函数的调用中缺少参数。应该在函数名后加上括号并传入一个Person类型的参数,例如printPerson(p)。
以下是修正后的代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Person {
int id;
string name;
int age;
char gender;
};
vector<Person> persons = {
{1, "Tom", 20, 'M'},
{2, "Lucy", 25, 'F'},
{3, "Bob", 30, 'M'},
};
void printPerson(Person p) {
cout << p.id << "\t" << p.name << "\t" << p.age << "\t" << p.gender << endl;
}
void queryByName(string name) {
bool found = false;
for (auto& p : persons) {
if (p.name == name) {
printPerson(p);
found = true;
}
}
if (!found) {
cout << "No person with that name." << endl;
}
}
int main() {
cout << "ID\tName\tAge\tGender" << endl;
for (auto& p : persons) {
printPerson(p);
}
string name;
cout << "Enter a name to query: ";
cin >> name;
queryByName(name);
return 0;
}
```
NormalMilitiaman nm = queryByName(name);
根据您的代码推测,您可能是在使用 Mybatis-Plus 进行数据库操作。`queryByName(name)` 可能是您自己定义的查询方法,用于根据名称查询数据表中的某一条记录,并将查询结果封装为 `NormalMilitiaman` 对象返回。
如果您使用了 Mybatis-Plus,可以通过继承 `BaseMapper` 接口并编写自己的方法来实现数据的增删改查操作。假设您的数据表对应的实体类为 `NormalMilitiaman`,您可以编写如下代码来实现根据名称查询一条记录的功能:
```java
public interface NormalMilitiamanMapper extends BaseMapper<NormalMilitiaman> {
NormalMilitiaman queryByName(@Param("name") String name);
}
```
其中,`@Param("name")` 注解用于指定方法参数名称,在 SQL 语句中使用参数时需要使用该名称。具体的 SQL 语句可以在 XML 配置文件中编写,例如:
```xml
<select id="queryByName" resultType="com.example.entity.NormalMilitiaman">
SELECT * FROM normal_militiaman WHERE name = #{name}
</select>
```
这样,您就可以在需要查询名称为 `name` 的记录时调用 `queryByName(name)` 方法来获取结果了。
阅读全文
相关推荐
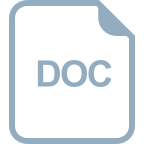
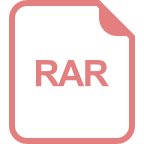













