python爬虫 多线程
时间: 2023-11-08 21:48:46 浏览: 93
Python爬虫的多线程可以通过多种方式实现,其中比较常用的是使用`threading`模块或`concurrent.futures`模块。
使用`threading`模块实现多线程爬虫的基本步骤如下:
1. 定义一个爬取任务的函数
2. 创建多个线程,每个线程执行爬取任务函数
3. 启动线程,等待线程执行完毕
示例代码如下:
```python
import threading
import requests
def crawl(url):
# 爬取任务函数
response = requests.get(url)
print(response.text)
urls = ['http://www.baidu.com', 'http://www.taobao.com', 'http://www.jd.com']
threads = []
for url in urls:
thread = threading.Thread(target=crawl, args=(url,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
使用`concurrent.futures`模块实现多线程爬虫的基本步骤如下:
1. 定义一个爬取任务的函数
2. 创建线程池对象
3. 提交任务到线程池,返回`Future`对象
4. 获取`Future`对象的结果
示例代码如下:
```python
import concurrent.futures
import requests
def crawl(url):
# 爬取任务函数
response = requests.get(url)
return response.text
urls = ['http://www.baidu.com', 'http://www.taobao.com', 'http://www.jd.com']
with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(crawl, url) for url in urls]
for future in concurrent.futures.as_completed(futures):
print(future.result())
```
以上是两种常用的Python爬虫多线程实现方式,可以根据实际需求选择适合的方法。
阅读全文
相关推荐
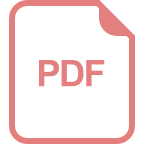
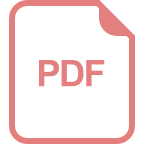
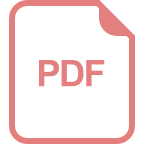














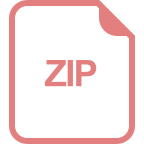
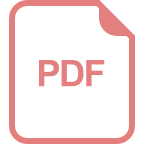