time_time与time_ctime的区别
时间: 2023-07-19 07:53:42 浏览: 44
`time_time` 和 `time_ctime` 都是 Python 中的时间处理函数,它们的主要区别在于返回值的格式不同。
`time_time()` 函数返回从 1970 年 1 月 1 日午夜 UTC(协调世界时)到现在经过的秒数,即时间戳。时间戳是一个浮点数,可以用来进行各种时间计算。
`time_ctime()` 函数接收一个时间戳作为参数,并返回一个字符串,表示该时间戳对应的本地时间。字符串的格式为 "Sun May 23 12:14:36 2021"。
因此,如果你只需要获取当前时间戳,可以使用 `time_time()` 函数。如果你需要将时间戳转换为字符串格式,可以使用 `time_ctime()` 函数。
相关问题
#define+_TIME_
根据提供的引用内容,#define _TIME_并不是一个常见的宏定义。但是,我们可以使用#define指令来定义一个名为_TIME_的宏。该宏可以用于在程序中引用当前系统时间。下面是一个示例代码:
```c
#include <stdio.h>
#include <time.h>
#define _TIME_ time(NULL)
int main() {
printf("Current time: %s", ctime(&_TIME_));
return 0;
}
```
在上面的代码中,我们使用#define指令定义了一个名为_TIME_的宏,该宏使用time(NULL)函数获取当前系统时间。然后,我们在程序中使用_TIME_宏来引用当前时间,并使用ctime()函数将其转换为可读的格式进行输出。
time_print
time_print是一个自定义的函数,用于打印当前的时间。它可以通过以下步骤来实现:
1. 获取当前的时间戳。
2. 将时间戳转换为可读的时间格式。
3. 打印出转换后的时间。
以下是一个示例的time_print函数的实现:
```python
import time
def time_print():
current_time = time.time()
readable_time = time.ctime(current_time)
print("Current time is:", readable_time)
```
调用time_print函数后,它会打印出当前的时间。你可以根据自己的需求对该函数进行修改和扩展。
相关推荐
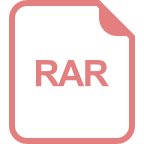
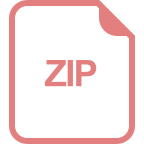
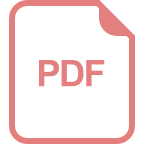












