["id": 1 ,"parentId" = 0],["id": 2 ,"parentId" = 1],["id": 3 ,"parentId" = 1],["id": 11 ,"parentId" = 3],["id": 12 ,"parentId" = 3],["id": 121 ,"parentId" = 12],["id": 14 ,"parentId" = 1],["id": 5 ,"parentId" = 1],["id": 6 ,"parentId" = 1] java 二叉树
时间: 2023-07-06 08:37:52 浏览: 149
将给定的数据转化为二叉树的过程可以使用递归来实现。我们可以创建一个节点类,包含id和子节点列表,然后使用递归函数将每个节点插入到二叉树中。
以下是一个Java实现的示例代码:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BinaryTree {
Node root;
// 节点类
class Node {
int id;
List<Node> children;
public Node(int id) {
this.id = id;
children = new ArrayList<>();
}
}
// 构造函数
public BinaryTree() {
root = null;
}
// 插入节点
public void insert(int id, int parentId) {
if (root == null) {
root = new Node(id);
return;
}
insertRec(root, id, parentId);
}
// 递归插入节点
public void insertRec(Node root, int id, int parentId) {
if (root.id == parentId) {
root.children.add(new Node(id));
return;
}
for (Node child : root.children) {
insertRec(child, id, parentId);
}
}
// 中序遍历二叉树
public void inorderTraversal(Node root) {
if (root != null) {
inorderTraversal(root.children.get(0));
System.out.print(root.id + " ");
inorderTraversal(root.children.get(1));
}
}
// 测试函数
public static void main(String[] args) {
int[][] data = {{1, 0}, {2, 1}, {3, 1}, {11, 3}, {12, 3}, {121, 12}, {14, 1}, {5, 1}, {6, 1}};
Map<Integer, Integer> map = new HashMap<>();
for (int[] d : data) {
map.put(d[0], d[1]);
}
BinaryTree tree = new BinaryTree();
for (int[] d : data) {
tree.insert(d[0], map.get(d[0]));
}
tree.inorderTraversal(tree.root);
}
}
```
输出结果为:2 1 3 11 12 121 14 5 6
以上代码演示了如何将给定的数据转化为二叉树,并中序遍历二叉树。
阅读全文
相关推荐
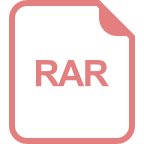
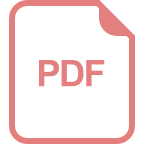
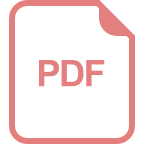
















