java 使用opencv 计算2张图片的相似度
时间: 2023-10-27 13:07:10 浏览: 52
要使用Java和OpenCV计算两张图片的相似度,可以采用以下步骤:
1. 加载图片:使用OpenCV的Java接口,加载两张待比较的图片,可以使用Imgcodecs.imread方法读取图片。
2. 提取特征:使用OpenCV的FeatureDetector和DescriptorExtractor等类,对图片进行特征提取和描述。
3. 计算相似度:使用OpenCV的DescriptorMatcher类,将提取的特征进行匹配,并计算相似度。
下面是一个简单的Java代码示例:
```
import org.opencv.core.*;
import org.opencv.features2d.*;
import org.opencv.imgcodecs.Imgcodecs;
public class ImageMatcher {
public static void main(String[] args) {
// 加载图片
Mat img1 = Imgcodecs.imread("image1.jpg");
Mat img2 = Imgcodecs.imread("image2.jpg");
// 提取ORB特征
ORB orb = ORB.create();
MatOfKeyPoint keypoints1 = new MatOfKeyPoint();
MatOfKeyPoint keypoints2 = new MatOfKeyPoint();
Mat descriptors1 = new Mat();
Mat descriptors2 = new Mat();
orb.detectAndCompute(img1, new Mat(), keypoints1, descriptors1);
orb.detectAndCompute(img2, new Mat(), keypoints2, descriptors2);
// 计算相似度
DescriptorMatcher matcher = DescriptorMatcher.create(DescriptorMatcher.BRUTEFORCE_HAMMING);
MatOfDMatch matches = new MatOfDMatch();
matcher.match(descriptors1, descriptors2, matches);
// 输出相似度
double maxDist = 0;
double minDist = 100;
DMatch[] matchArray = matches.toArray();
for (int i = 0; i < matchArray.length; i++) {
double dist = matchArray[i].distance;
if (dist < minDist) minDist = dist;
if (dist > maxDist) maxDist = dist;
}
double similarity = (1 - minDist / maxDist) * 100;
System.out.println("相似度:" + similarity + "%");
}
}
```
在这个例子中,我们使用了OpenCV的ORB算法进行特征提取,使用了Hamming距离进行特征匹配,计算出了两张图片的相似度。请注意,在实际应用中可能需要根据具体情况选择不同的算法和参数,以获得更准确的结果。
相关推荐
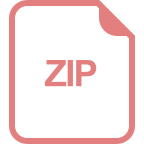
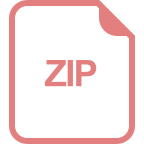














