写一个网络爬虫的代码并注释
时间: 2023-09-05 21:04:45 浏览: 60
### 回答1:
示例代码:# 导入所需模块
import requests
from bs4 import BeautifulSoup# 获取网页
url = "https://www.example.com/"
page = requests.get(url)# 解析网页
soup = BeautifulSoup(page.text, 'html.parser')# 查找所需的内容
content = soup.find('div', class_='content')# 打印所查找的内容
print(content)
### 回答2:
下面是一个编写网络爬虫的示例代码:
```python
import requests # 导入requests模块,用于发送HTTP请求
from bs4 import BeautifulSoup # 导入BeautifulSoup模块,用于解析HTML页面
# 定义一个函数,用于获取指定URL的页面内容
def get_html(url):
response = requests.get(url) # 发送GET请求获取页面内容
if response.status_code == 200: # 如果返回状态码为200,表示请求成功
return response.text # 返回页面内容
else:
return None # 请求失败,返回None
# 定义一个函数,用于解析HTML页面并提取所需信息
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser') # 解析HTML页面
# 在这里编写具体的解析代码,提取所需信息
# 这里以提取页面中所有的链接为例
links = soup.find_all('a') # 使用find_all方法找到所有<a>标签
for link in links:
print(link['href']) # 打印链接的href属性值
def main():
url = 'https://www.example.com' # 定义要爬取的URL
html = get_html(url) # 获取页面内容
if html:
parse_html(html) # 解析页面并提取信息
if __name__ == '__main__':
main()
```
上述代码是一个简单的网络爬虫示例,使用了Python中的requests和BeautifulSoup模块。首先,通过get_html函数发送GET请求获取页面内容。接着,通过parse_html函数解析HTML页面并提取所需信息。以上述代码为例,演示了如何提取页面中所有的链接。最后,在main函数中调用上述两个函数实现网络爬取的整个流程。
### 回答3:
下面是一个简单的网络爬虫代码的示例,并且添加了一些注释说明:
```python
import requests # 导入用于发送HTTP请求的库
from bs4 import BeautifulSoup # 导入用于解析HTML的库
def get_html(url):
# 发送GET请求并获取网页内容
response = requests.get(url)
# 将内容转换为文本格式
html = response.text
return html
def parse_html(html):
# 使用BeautifulSoup库解析HTML
soup = BeautifulSoup(html, 'html.parser')
# 通过选择器定位需要提取的内容,这里以提取标题为例
titles = soup.select('h1.title')
# 遍历标题并打印
for title in titles:
print(title.text)
def main():
# 要爬取的网页URL
url = 'http://example.com'
# 获取网页内容
html = get_html(url)
# 解析网页并提取需要的内容
parse_html(html)
if __name__ == "__main__":
main()
```
这个示例中,我们首先导入了需要的库。然后定义了两个函数,`get_html`用于发送GET请求并获取网页内容,`parse_html`用于解析HTML并提取需要的内容。在`parse_html`中,我们使用BeautifulSoup库对HTML进行解析,并通过选择器定位需要的标题。最后,在`main`函数中,我们指定要爬取的网页URL,然后调用前面定义的函数来执行爬取操作。
相关推荐
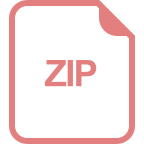
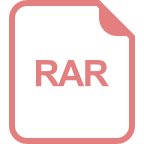
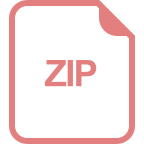
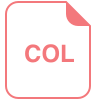













