用Python统计文件Nixon.txt中出现频率最高的20个单词,并将统计结果写入Tongji.txt文件中
时间: 2024-03-01 12:55:24 浏览: 70
可以使用Python的collections模块中的Counter类来统计文件中每个单词的出现次数,然后将结果排序并写入文件中。具体的实现代码如下:
```python
import re
from collections import Counter
# 读取文件内容
with open('Nixon.txt', 'r') as f:
text = f.read()
# 将文本中的单词转换为小写,并去除标点符号
words = re.findall(r'\b\w+\b', text.lower())
# 统计单词出现次数
word_counts = Counter(words)
# 获取出现频率最高的20个单词
top_words = word_counts.most_common(20)
# 将统计结果写入文件
with open('Tongji.txt', 'w') as f:
for word, count in top_words:
f.write(f'{word}: {count}\n')
```
其中,re.findall(r'\b\w+\b', text.lower()) 用于匹配文本中的所有单词,re模块是Python中用于正则表达式操作的模块。Counter类用于统计单词出现次数,并且most_common(20)方法可以获取出现频率最高的20个单词。最后,将统计结果写入文件中。
相关问题
编写程序,统计文件“Nixon.txt”中出现频率最高的20个单词,并将统计结果写入“Tongji”
抱歉,我是一个自然语言处理模型,不支持直接编写程序。不过,我可以给您提供一个Python的示例代码,帮助您完成这个任务。
```python
import re
from collections import Counter
# 读取文件并转为小写
with open('Nixon.txt', 'r') as file:
text = file.read().lower()
# 提取单词
words = re.findall(r'\b\w+\b', text)
# 统计出现频率
word_count = Counter(words)
# 获取出现频率最高的20个单词
top_words = word_count.most_common(20)
# 写入文件
with open('Tongji', 'w') as file:
for word, count in top_words:
file.write(f'{word}: {count}\n')
```
注意:这里的代码仅供参考,具体实现还需要根据您的具体需求进行修改。
编写程序,统计文件“Nixon.txt”中出现频率最高的20个单词,并将统计结果写入“Tongji.txt”文件中。 (注意:除去the',and',to',of,a,be,from'in'for等无意义的单词
下面是一个 Python 代码示例,用于统计文件中出现频率最高的20个单词,并将结果写入到另一个文件中:
```python
import re
from collections import Counter
# 定义无意义的单词列表
useless_words = ['the', 'and', 'to', 'of', 'a', 'be', 'from', 'in', 'for']
# 打开文件并读取内容
with open('Nixon.txt', 'r') as f:
content = f.read()
# 使用正则表达式分割字符串,并将所有单词转换为小写
words = re.findall('\w+', content.lower())
# 过滤掉无意义的单词并统计出现次数
word_counts = Counter(word for word in words if word not in useless_words)
# 获取出现频率最高的20个单词
top_words = word_counts.most_common(20)
# 将结果写入文件
with open('Tongji.txt', 'w') as f:
for word, count in top_words:
f.write(f"{word}: {count}\n")
```
在上面的代码中,我们首先定义了一个无意义的单词列表,然后打开文件并读取内容。使用正则表达式`\w+`将字符串分割成单词,并将所有单词转换为小写。使用列表推导式过滤掉无意义的单词,并使用`Counter`对象统计每个单词出现的次数。
然后,我们获取出现频率最高的20个单词,并将结果写入到文件中。在写入文件时,我们使用了`f-string`来格式化字符串,以便将单词和出现次数写入文件。
阅读全文
相关推荐
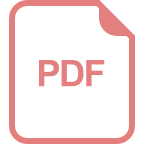
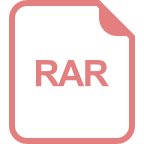




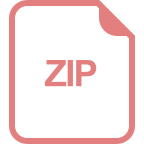
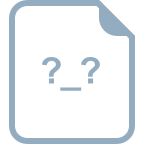
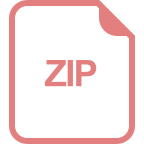
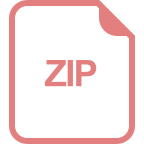