假设你是一个python程序员,现在你需要创建两个脚本,脚本A通过一个线程函数,启动tcp的异步客户端,这个客户端首先需要和服务端建立连接,脚本内有一个连接成功的变量,如果建立成功变量为真反之为假,同时该脚本还有一个专门与服务端发送消息的函数,脚本B可以通过这个函数向服务端发送内容
时间: 2023-08-08 14:07:14 浏览: 80
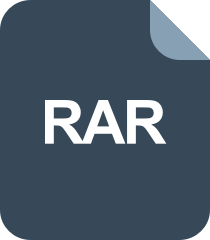
python实现tcp服务器和客户端
好的,我可以为您提供这两个脚本的示例代码。首先是脚本A:
```python
import asyncio
import threading
connected = False
async def tcp_client():
global connected
reader, writer = await asyncio.open_connection('localhost', 8888)
connected = True
while True:
data = await reader.read(4096)
if not data:
break
print(f'Received: {data.decode()}')
writer.close()
def start_client():
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
loop.run_until_complete(tcp_client())
thread = threading.Thread(target=start_client)
thread.start()
```
这个脚本首先定义了一个变量`connected`,用于保存连接状态。`tcp_client()`函数是一个异步函数,用于启动异步TCP客户端并连接到指定的服务端。这个函数中会一直读取服务端发送来的数据,并输出到控制台。
`start_client()`函数用于在一个新线程中启动`tcp_client()`函数。最后一行代码会启动这个线程。
接下来是脚本B,用于向服务端发送消息:
```python
import asyncio
async def send_message(message):
while not connected:
await asyncio.sleep(0.1)
writer.write(message.encode())
await writer.drain()
print(f'Sent: {message}')
async def run():
await send_message('Hello, world!')
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
if not connected:
loop.run_until_complete(tcp_client())
reader, writer = loop.run_until_complete(asyncio.open_connection('localhost', 8888))
loop.run_until_complete(run())
```
这个脚本导入了之前定义的`connected`变量,并定义了一个异步函数`send_message()`,用于向服务端发送消息。这个函数会等待连接成功后再发送消息。
`run()`函数是一个异步函数,用于调用`send_message()`函数并发送一条消息。
最后,创建一个新的事件循环并在其中运行`run()`函数,就可以向服务端发送一条消息了。
阅读全文
相关推荐
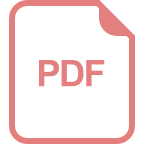
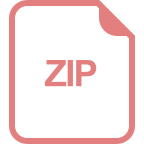
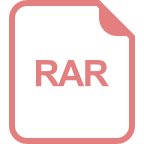
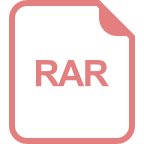
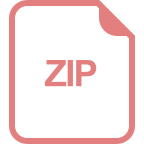
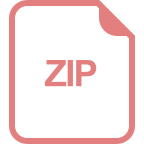
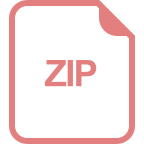
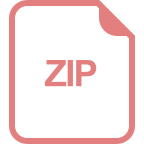
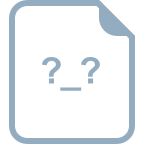
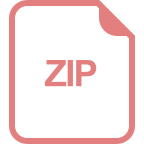
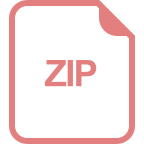
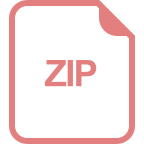
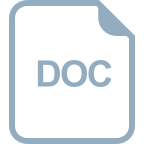
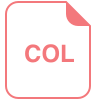
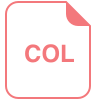
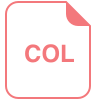
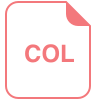
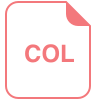