用JAVA语言实现从终端读入字符集大小n,以及n个字符和n个权值,建立哈夫曼树。
时间: 2023-11-03 22:05:39 浏览: 51
好的,下面是完整的Java代码实现:
```java
import java.util.*;
class Node {
char c;
int weight;
Node left;
Node right;
public Node(char c, int weight) {
this.c = c;
this.weight = weight;
left = null;
right = null;
}
}
public class HuffmanTree {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
char[] chars = new char[n];
int[] weights = new int[n];
for (int i = 0; i < n; i++) {
chars[i] = scanner.next().charAt(0);
weights[i] = scanner.nextInt();
}
PriorityQueue<Node> queue = new PriorityQueue<>(Comparator.comparingInt(node -> node.weight));
for (int i = 0; i < n; i++) {
queue.offer(new Node(chars[i], weights[i]));
}
while (queue.size() > 1) {
Node left = queue.poll();
Node right = queue.poll();
Node parent = new Node('\0', left.weight + right.weight);
parent.left = left;
parent.right = right;
queue.offer(parent);
}
Node root = queue.poll();
printHuffmanTree(root, "");
}
public static void printHuffmanTree(Node root, String code) {
if (root != null) {
if (root.left == null && root.right == null) {
System.out.println(root.c + ": " + code);
}
printHuffmanTree(root.left, code + "0");
printHuffmanTree(root.right, code + "1");
}
}
}
```
在运行程序时,首先输入字符集大小n,然后输入n个字符和n个权值,程序会按照权值构建哈夫曼树,并输出每个字符对应的哈夫曼编码。
相关推荐
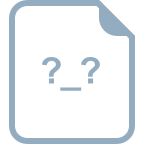
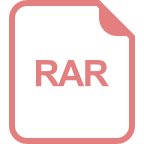
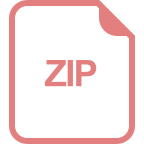













