用C++实现opencv调用摄像头
时间: 2024-05-19 13:15:51 浏览: 72
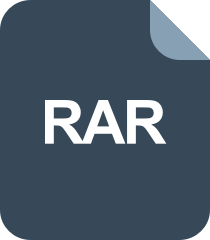
基于opencv调出摄像头
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 打开默认摄像头
VideoCapture cap(0);
// 检查是否成功打开摄像头
if (!cap.isOpened()) {
cerr << "Unable to open the default camera!" << endl;
return -1;
}
// 创建窗口
namedWindow("Camera", WINDOW_AUTOSIZE);
while (true) {
Mat frame;
// 读取一帧图像
cap.read(frame);
// 显示图像
imshow("Camera", frame);
// 等待按键
int key = waitKey(30);
// 按下ESC键退出
if (key == 27) {
break;
}
}
// 释放摄像头
cap.release();
// 关闭窗口
destroyAllWindows();
return 0;
}
阅读全文
相关推荐
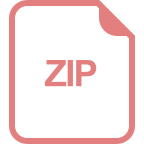
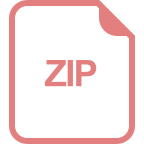
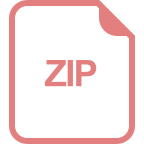




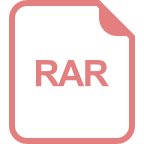
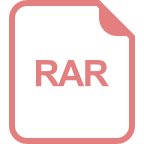
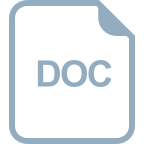



