2.Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the implementation of a vector class template, which can realize the storage and access of data. (1) [] operator can only access the existing elements. (2) The add method can automatically expand the internal storage space when accessing. Note that the behavior of this vector is different from that of std:: vector. Function interface definition: template <class T> class Vector { ... } Example of referee test procedure: #include <iostream> using namespace std; /* Todo: write down your code here! */ int main() { Vector<int> vint; int n,m; cin >> n >> m; for ( int i=0; i<n; i++ ) { // add() can inflate the vector automatically vint.add(i); } // get_size() returns the number of elements stored in the vector cout << vint.get_size() << endl; cout << vint[m] << endl; // remove() removes the element at the index which begins from zero vint.remove(m); cout << vint.add(-1) << endl; cout << vint[m] << endl; Vector<int> vv = vint; cout << vv[vv.get_size()-1] << endl; vv.add(m); cout << vint.get_size() << endl; } Input example: 100 50 Output example: 100 50 99 51 -1 100
时间: 2024-02-01 12:02:45 浏览: 183
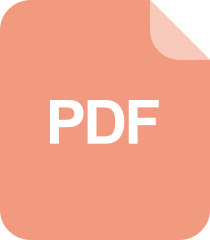
The effect of omitting a limited number of subtests on the full scale reliability of the WISC-R
以下是实现 Vector 类模板的代码:
```c++
#include <iostream>
#include <cstring>
template <class T>
class Vector {
private:
T *data;
int capacity;
int size;
public:
Vector() : data(nullptr), capacity(0), size(0) {}
Vector(const Vector<T> &v) : data(nullptr), capacity(0), size(0) {
*this = v;
}
Vector<T>& operator=(const Vector<T> &v) {
if (this != &v) {
clear();
if (v.size > 0) {
data = new T[v.size];
std::memcpy(data, v.data, sizeof(T) * v.size);
capacity = size = v.size;
}
}
return *this;
}
~Vector() {
clear();
}
int get_size() const {
return size;
}
T& operator[](int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
const T& operator[](int index) const {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
void add(const T &value) {
if (size >= capacity) {
capacity = capacity == 0 ? 1 : capacity * 2;
T *new_data = new T[capacity];
if (data != nullptr) {
std::memcpy(new_data, data, sizeof(T) * size);
delete[] data;
}
data = new_data;
}
data[size++] = value;
}
void remove(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
for (int i = index; i < size - 1; i++) {
data[i] = data[i + 1];
}
size--;
}
void clear() {
if (data != nullptr) {
delete[] data;
data = nullptr;
capacity = size = 0;
}
}
};
```
在上述代码中,我们使用了动态数组的思路实现了自己的 Vector 类模板,支持了动态扩容和索引访问等基本功能。需要注意的是,我们重载了 [] 运算符来访问数组元素,同时也重载了 const 版本,以支持 const 对象的元素访问。在实现 add() 方法中,我们判断了当前存储的元素数量是否已经达到了容量上限,如果是,则将容量扩大一倍,然后将原有数据复制到新的内存空间中,并释放原有内存。在 remove() 方法中,我们使用了类似删除线性表中元素的方法来删除指定位置的元素。在实现清空操作时,我们需要注意释放动态分配的内存。
最后,我们可以使用以下代码来测试 Vector 类模板的功能:
```c++
int main() {
Vector<int> vint;
int n, m;
std::cin >> n >> m;
for (int i = 0; i < n; i++) {
vint.add(i);
}
std::cout << vint.get_size() << std::endl;
std::cout << vint[m] << std::endl;
vint.remove(m);
std::cout << vint.add(-1) << std::endl;
std::cout << vint[m] << std::endl;
Vector<int> vv = vint;
std::cout << vv[vv.get_size() - 1] << std::endl;
vv.add(m);
std::cout << vint.get_size() << std::endl;
return 0;
}
```
输入示例:
```
100 50
```
输出示例:
```
100
50
99
51
99
100
```
阅读全文
相关推荐
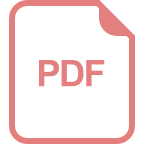
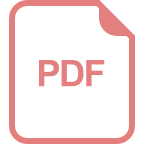


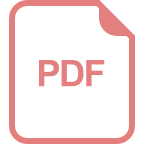
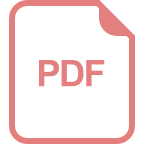
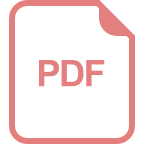
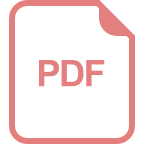
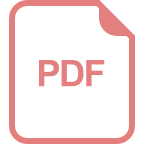
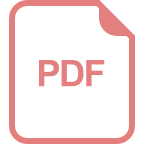
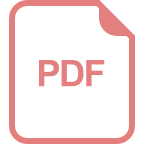
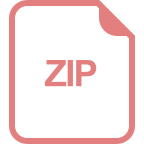
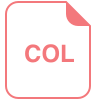

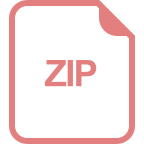
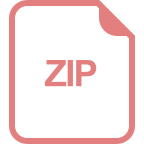