定义一个学生结构体,其中有5个数据成员有学号、姓名、数学成绩、外语成绩,语文成绩。编写主函数使用这个结构体,实现对学生数据的赋值和输出。实现求出总分最高学生的学号与姓名。采用结构体存储数据,输入数据时,要求学号不能相同,姓名可以相同。
时间: 2023-12-06 15:42:15 浏览: 47
以下是该题的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
string id; // 学号
string name; // 姓名
int mathScore; // 数学成绩
int englishScore; // 外语成绩
int chineseScore; // 语文成绩
};
int main() {
const int numStudents = 3; // 学生人数
Student students[numStudents];
int totalScoreMax = -1; // 最高总分
string idMax, nameMax; // 总分最高学生的学号和姓名
// 输入学生信息
for (int i = 0; i < numStudents; i++) {
cout << "请输入第" << i + 1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> students[i].id;
// 判断学号是否已存在
for (int j = 0; j < i; j++) {
if (students[i].id == students[j].id) {
cout << "学号已存在,请重新输入。" << endl;
i--;
break;
}
}
cout << "姓名:";
cin >> students[i].name;
cout << "数学成绩:";
cin >> students[i].mathScore;
cout << "外语成绩:";
cin >> students[i].englishScore;
cout << "语文成绩:";
cin >> students[i].chineseScore;
cout << endl;
}
// 输出学生信息
cout << "学生信息如下:" << endl;
for (int i = 0; i < numStudents; i++) {
cout << "学号:" << students[i].id << endl;
cout << "姓名:" << students[i].name << endl;
cout << "数学成绩:" << students[i].mathScore << endl;
cout << "外语成绩:" << students[i].englishScore << endl;
cout << "语文成绩:" << students[i].chineseScore << endl;
cout << endl;
// 计算总分
int totalScore = students[i].mathScore + students[i].englishScore + students[i].chineseScore;
if (totalScore > totalScoreMax) {
totalScoreMax = totalScore;
idMax = students[i].id;
nameMax = students[i].name;
}
}
// 输出总分最高学生的学号和姓名
cout << "总分最高学生的学号和姓名为:" << idMax << " " << nameMax << endl;
return 0;
}
```
以上代码实现了对学生信息的输入、输出以及求出总分最高学生的学号和姓名。在输入学号时,使用了一个循环来判断学号是否已经存在,如果已经存在则要求重新输入。
相关推荐
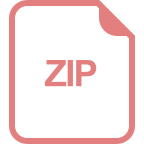
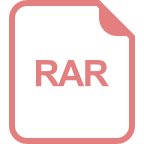
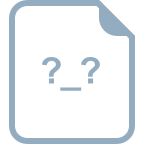
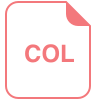
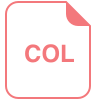
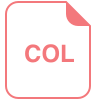
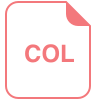
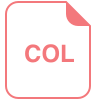









