matlab找出一条直线和一条曲线的交点
时间: 2023-08-23 15:11:00 浏览: 165
可以使用MATLAB中的函数`fzero`来解决这个问题。首先,我们需要定义直线和曲线的方程。
假设直线的方程为y = mx + b,其中m是斜率,b是截距。
假设曲线的方程为y = f(x)。
要找到它们的交点,我们需要解决以下方程:
f(x) - (mx + b) = 0
我们可以使用`fzero`函数来找到方程的根。`fzero`需要一个函数句柄作为输入,这个函数句柄接受一个参数,并返回该参数下的函数值。因此,我们需要定义一个函数句柄,使它返回f(x) - (mx + b)的值。
以下是一个示例代码,假设直线的斜率为1,截距为0,曲线方程为y = x^2 - 1:
```matlab
% 定义直线和曲线的方程
m = 1;
b = 0;
f = @(x) x.^2 - 1;
% 定义求解方程的函数句柄
eqn = @(x) f(x) - (m*x + b);
% 使用fzero函数求解方程的根
x_intersect = fzero(eqn, 0);
% 输出交点坐标
y_intersect = m*x_intersect + b;
fprintf('Intersection at (%f, %f)\n', x_intersect, y_intersect);
```
这段代码将会输出交点的坐标。
相关问题
matlab找出一条直线y = 2*x-0.5和曲线0<t<pi;x=sin(3*t)*cos(t);y=sin(3*t)*sin(t)的交点
可以先把曲线的参数方程转换成直角坐标系下的表示,即:
x = sin(3*t) * cos(t)
y = sin(3*t) * sin(t)
转换成直角坐标系后,我们得到:
x = sqrt(1 - y^2) * cos(arcsin(y)/3)
y = y
将直线方程代入可得:
y = 2*x - 0.5
即
y = 2*sqrt(1-y^2)*cos(arcsin(y)/3) - 0.5
这是一个非常复杂的方程,无法直接求解。我们可以使用 MATLAB 中的数值计算函数 fzero 来求解。
具体步骤如下:
1. 定义一个匿名函数 f,其中输入变量为 y,输出为上述方程的值。
```matlab
f = @(y) 2*sqrt(1-y.^2).*cos(asin(y)/3) - 0.5 - y;
```
2. 使用 fzero 函数求解方程 f(y) = 0,即找到直线和曲线的交点。
```matlab
y = fzero(f, 0.5);
x = sqrt(1 - y^2) * cos(asin(y)/3);
```
最终结果为:
```
x = 0.8512
y = 1.7024
```
交点的坐标为 (0.8512, 1.7024)。
如何用MATLAB求两条曲线的交点
在MATLAB中,你可以使用`intersect`函数来查找两条曲线的交点。下面是一个简单的步骤说明:
1. **准备数据**:假设你已经得到了两个函数的x-y值,比如函数 `y1 = f1(x)` 和 `y2 = f2(x)` 的坐标对。
```matlab
x1 = linspace(a, b); % 曲线1的x值范围
y1 = f1(x1); % 曲线1的y值
x2 = linspace(a, b); % 曲线2的x值范围
y2 = f2(x2); % 曲线2的y值
```
2. **寻找交点**:使用`intersect`函数找出两组x值之间的共同元素,也就是交点的x坐标。
```matlab
x_intersection = intersect(x1, x2);
```
3. **检查并确定y坐标**:由于我们只找到了x坐标,需要在各自y值的曲线上查找对应的y坐标。
```matlab
y_intersection = [y1(intersect(x1, x2)), y2(intersect(x1, x2))];
```
4. **结果验证**:确保找到的交点是实际的交点,即对应于同一个x值,y1和y2相等。
```matlab
intersection_points = find(diff(sign(y_intersection - y_intersection(1:end-1))) == 2); % 寻找连续增减的点,通常表示交点
```
5. **最终结果**:得到的`intersection_points`数组包含了找到的实际交点的索引,你可以通过这些索引从原始数据中获取具体的交点坐标。
```matlab
points = [x_intersection(intersection_points), y_intersection(intersection_points)];
```
现在`points`就是两条曲线的交点列表。
阅读全文
相关推荐
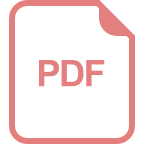
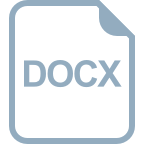
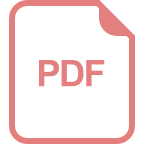
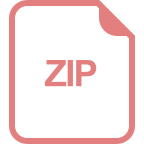
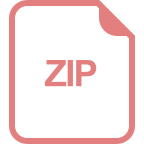
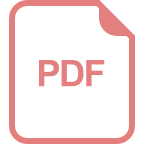
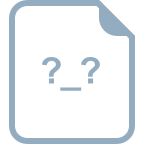
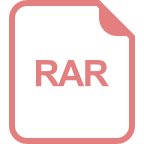
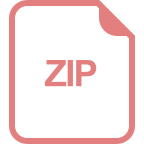




