a星算法求解8数码问题的python源代码
时间: 2024-11-12 13:33:03 浏览: 74
A*(A-star)算法是一种启发式搜索算法,用于解决像迷宫寻找最短路径、八皇后问题等优化问题。对于八数码问题(也称作数独),虽然直接应用A*不是标准做法,因为数独有明确的规则和步进策略,通常使用回溯法(Backtracking)更为合适。不过,如果你想用A*算法作为启发式搜索的一个例子,你可以将它作为一个通用的框架,并结合数独的特点进行调整。
以下是简单的Python实现思路,用于演示如何使用A*算法求解一般的状态空间搜索,实际处理八数码问题需要额外的转换和状态检查:
```python
import heapq
def heuristic(state, goal):
# 这里只是一个简化版的启发函数,对于数独,可以用未填数字的数量作为代价
return len([cell for row in state for cell in row if cell == 0])
def a_star_search(start, goal):
frontier = [(0, start)]
came_from = {}
cost_so_far = {start: 0}
while frontier:
_, current = heapq.heappop(frontier)
if current == goal:
break
for neighbor, new_cost in generate_neighbors(current):
old_cost = cost_so_far.get(neighbor, float('inf'))
new_cost += new_heuristic(neighbor, goal)
if new_cost < old_cost:
cost_so_far[neighbor] = new_cost
priority = new_cost + heuristic(neighbor, goal)
heapq.heappush(frontier, (priority, neighbor))
came_from[neighbor] = current
path = []
while current != start:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
# 模拟生成邻接节点的方法,你需要根据实际情况替换
def generate_neighbors(state):
# 对于数独,这会是一个更复杂的函数,考虑行、列、宫格的唯一值
pass
# 仅适用于简单情况的启发函数
def new_heuristic(state, goal):
return heuristic(state, goal)
# 初始化开始状态(这里假设已知)
start_state = ... # 数独的初始空白布局
goal_state = ... # 解决后的状态
solution_path = a_star_search(start_state, goal_state)
```
请注意,这个代码并没有直接处理数独游戏,而是展示了A*算法的基本结构。在实际应用中,你需要针对数独特性和规则进行相应的修改。
阅读全文
相关推荐
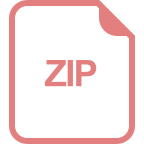
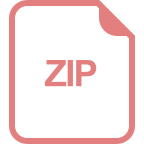
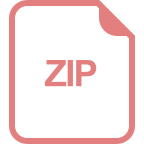
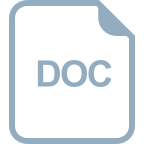
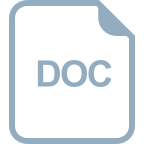
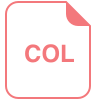
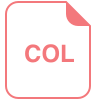
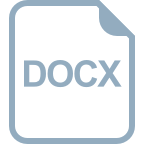
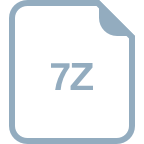
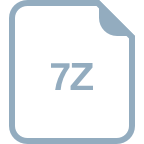
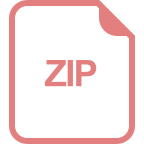
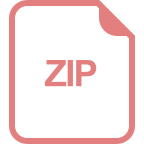
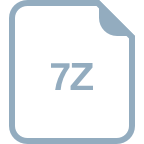
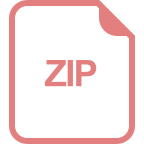