用html+JS代码写一个添加问题页面,页面分为左右两个盒子,在页面左侧盒子可以选择题型,能够实现添加单选题,多选题和问答题功能。页面右侧盒子有标题输入框、问卷说明输入框以及一个空白区域,使用左侧添加题型功能
时间: 2023-06-17 16:06:36 浏览: 110
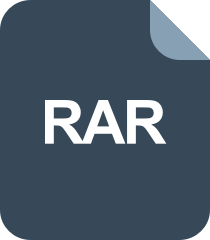
js页面中添加 ---左右添加多选框
可以参考以下代码实现:
```html
<!DOCTYPE html>
<html>
<head>
<title>添加问题页面</title>
<script>
// 定义题目类型枚举
var QuestionType = {
SINGLE_CHOICE: "单选题",
MULTIPLE_CHOICE: "多选题",
SHORT_ANSWER: "问答题"
};
// 定义题目对象
function Question(type, content, options) {
this.type = type;
this.content = content;
this.options = options;
}
// 初始化题目数组
var questions = [];
// 添加单选题
function addSingleChoice() {
var content = prompt("请输入题目内容:");
if (content) {
var optionCount = parseInt(prompt("请输入选项数量:"));
if (optionCount) {
var options = [];
for (var i = 0; i < optionCount; i++) {
options.push(prompt("请输入选项" + (i + 1) + ":"));
}
var question = new Question(QuestionType.SINGLE_CHOICE, content, options);
questions.push(question);
renderQuestions();
}
}
}
// 添加多选题
function addMultipleChoice() {
var content = prompt("请输入题目内容:");
if (content) {
var optionCount = parseInt(prompt("请输入选项数量:"));
if (optionCount) {
var options = [];
for (var i = 0; i < optionCount; i++) {
options.push(prompt("请输入选项" + (i + 1) + ":"));
}
var question = new Question(QuestionType.MULTIPLE_CHOICE, content, options);
questions.push(question);
renderQuestions();
}
}
}
// 添加问答题
function addShortAnswer() {
var content = prompt("请输入题目内容:");
if (content) {
var question = new Question(QuestionType.SHORT_ANSWER, content, null);
questions.push(question);
renderQuestions();
}
}
// 渲染题目列表
function renderQuestions() {
var questionList = document.getElementById("questionList");
questionList.innerHTML = "";
for (var i = 0; i < questions.length; i++) {
var question = questions[i];
var questionItem = document.createElement("div");
questionItem.className = "questionItem";
questionItem.innerHTML = "<span>" + question.type + "</span><span>" + question.content + "</span>";
questionList.appendChild(questionItem);
}
}
</script>
<style>
.container {
display: flex;
flex-direction: row;
height: 100vh;
}
.leftBox {
flex: 1;
padding: 20px;
background-color: #f0f0f0;
}
.rightBox {
flex: 3;
padding: 20px;
background-color: #fff;
}
.questionType {
margin-bottom: 20px;
}
.questionItem {
margin-bottom: 10px;
padding: 10px;
background-color: #f5f5f5;
border-radius: 5px;
}
</style>
</head>
<body>
<div class="container">
<div class="leftBox">
<div class="questionType">
<button onclick="addSingleChoice()">添加单选题</button>
</div>
<div class="questionType">
<button onclick="addMultipleChoice()">添加多选题</button>
</div>
<div class="questionType">
<button onclick="addShortAnswer()">添加问答题</button>
</div>
</div>
<div class="rightBox">
<div>
<label>标题:</label>
<input type="text">
</div>
<div>
<label>问卷说明:</label>
<textarea></textarea>
</div>
<div id="questionList"></div>
</div>
</div>
</body>
</html>
```
在页面左侧盒子中,分别添加了添加单选题、添加多选题和添加问答题的按钮。在点击按钮后,会弹出输入框,用户可以输入题目内容和选项,然后会将题目对象添加到题目数组中,并调用 `renderQuestions()` 函数重新渲染页面右侧盒子中的题目列表。
题目列表使用一个 `<div>` 元素来实现,每个题目使用一个 `<div>` 元素来表示,其中包含题目类型和题目内容两个 `<span>` 元素。
阅读全文
相关推荐
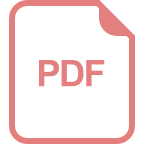

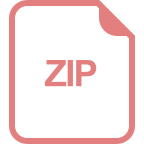
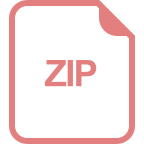
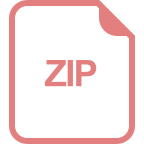



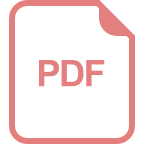
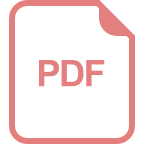
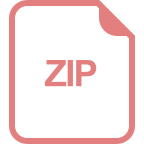
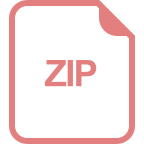
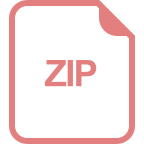