用C++定义一个基类车,包含轮子,长度,颜色,速度等基本数据成员,可通过构造 函数完成初始化;定义基类轮船,包含长度,高度,颜色,速度等基本数据成员, 通过构造函数完成初始化操作,定义派生类,水路两用汽车,具
时间: 2023-09-30 14:04:18 浏览: 68
有车和轮船的基本属性,可以在水上和陆地上行驶,同时还具有船只的浮力和推进性能。以下是代码实现:
```cpp
#include <iostream>
using namespace std;
class Vehicle {
protected:
int wheel_number;
int length;
string color;
int speed;
public:
Vehicle(int wheel_n, int l, string c, int s)
: wheel_number(wheel_n), length(l), color(c), speed(s) {}
void show() {
cout << "Wheel number: " << wheel_number << endl;
cout << "Length: " << length << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class Ship {
protected:
int length;
int height;
string color;
int speed;
public:
Ship(int l, int h, string c, int s)
: length(l), height(h), color(c), speed(s) {}
void show() {
cout << "Length: " << length << endl;
cout << "Height: " << height << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
class AmphibiousVehicle : public Vehicle, public Ship {
public:
AmphibiousVehicle(int wheel_n, int l, string c, int s, int h)
: Vehicle(wheel_n, l, c, s), Ship(l, h, c, s) {}
void show() {
cout << "Wheel number: " << wheel_number << endl;
cout << "Length: " << length << endl;
cout << "Height: " << height << endl;
cout << "Color: " << color << endl;
cout << "Speed: " << speed << endl;
}
};
int main() {
AmphibiousVehicle av(4, 10, "Blue", 60, 5);
av.show();
return 0;
}
```
在代码中,我们首先定义了基类 `Vehicle` 和 `Ship`,分别表示车和轮船。它们都有一些基本的属性,如长度、颜色、速度等。然后我们定义了派生类 `AmphibiousVehicle`,表示水路两用汽车,它同时具有车和轮船的属性,并且可以在陆地和水上行驶。在类的构造函数中,我们分别调用了基类 `Vehicle` 和 `Ship` 的构造函数来初始化它们的属性。最后,我们重写了 `show()` 函数来展示水路两用汽车的所有属性。在 `main()` 函数中,我们创建了一个 `AmphibiousVehicle` 对象,并调用了它的 `show()` 函数来展示它的属性。
阅读全文
相关推荐
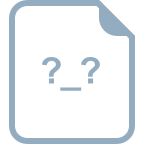
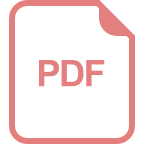
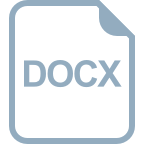
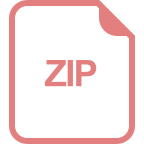
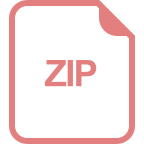
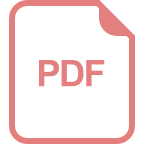
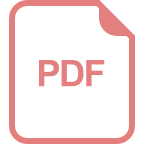
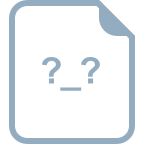
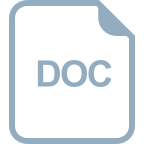
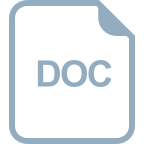
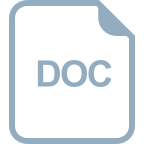
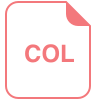
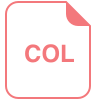
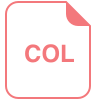
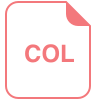
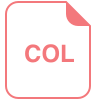
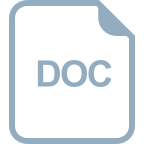