复数的加减运算(c++)
时间: 2023-07-06 12:04:48 浏览: 163
在C++中,复数可以使用结构体或者类来表示。以下是使用结构体实现复数加减运算的示例代码:
```c++
#include <iostream>
using namespace std;
struct Complex {
double real;
double imag;
};
Complex add(Complex c1, Complex c2) {
Complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
Complex sub(Complex c1, Complex c2) {
Complex result;
result.real = c1.real - c2.real;
result.imag = c1.imag - c2.imag;
return result;
}
int main() {
Complex c1, c2, res;
cout << "Enter the real and imaginary parts of first complex number: ";
cin >> c1.real >> c1.imag;
cout << "Enter the real and imaginary parts of second complex number: ";
cin >> c2.real >> c2.imag;
res = add(c1, c2);
cout << "Addition: " << res.real << " + " << res.imag << "i" << endl;
res = sub(c1, c2);
cout << "Subtraction: " << res.real << " + " << res.imag << "i" << endl;
return 0;
}
```
在上面的代码中,我们定义了一个复数结构体`Complex`,并实现了两个函数`add`和`sub`,分别用于计算复数的加法和减法。在`main`函数中,我们通过用户输入两个复数,调用`add`和`sub`函数进行计算,并输出结果。
另外,如果使用类来实现复数,可以将加法和减法运算符重载,使得代码更加简洁易读。以下是使用类实现复数加减运算的示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(Complex c2) {
return Complex(real + c2.real, imag + c2.imag);
}
Complex operator-(Complex c2) {
return Complex(real - c2.real, imag - c2.imag);
}
friend ostream& operator<<(ostream& os, Complex c) {
os << c.real << " + " << c.imag << "i";
return os;
}
private:
double real;
double imag;
};
int main() {
Complex c1, c2, res;
cout << "Enter the real and imaginary parts of first complex number: ";
cin >> c1;
cout << "Enter the real and imaginary parts of second complex number: ";
cin >> c2;
res = c1 + c2;
cout << "Addition: " << res << endl;
res = c1 - c2;
cout << "Subtraction: " << res << endl;
return 0;
}
```
在上面的代码中,我们定义了一个复数类`Complex`,其中重载了加法和减法运算符,并定义了一个友元函数重载输出运算符。在`main`函数中,我们通过用户输入两个复数,直接使用重载的运算符进行计算,并输出结果。
阅读全文
相关推荐
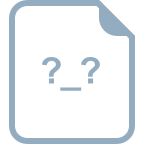

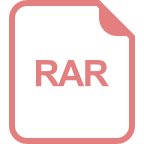
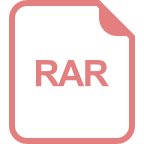
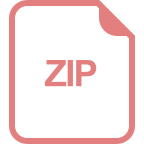
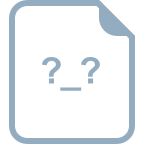
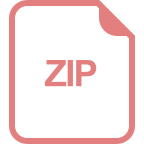
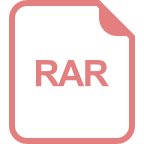
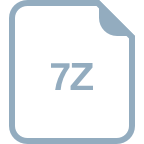
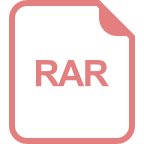
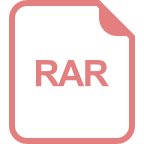




