复数加减乘除 C++
时间: 2023-06-29 17:21:06 浏览: 54
复数的加减乘除可以通过运算符重载来实现,下面是一个简单的复数类及其运算符重载实现:
```c++
#include <iostream>
class Complex {
public:
Complex(double real = 0.0, double imag = 0.0) : m_real(real), m_imag(imag) {}
Complex operator+(const Complex& other) const {
return Complex(m_real + other.m_real, m_imag + other.m_imag);
}
Complex operator-(const Complex& other) const {
return Complex(m_real - other.m_real, m_imag - other.m_imag);
}
Complex operator*(const Complex& other) const {
double real = m_real * other.m_real - m_imag * other.m_imag;
double imag = m_real * other.m_imag + m_imag * other.m_real;
return Complex(real, imag);
}
Complex operator/(const Complex& other) const {
double denominator = other.m_real * other.m_real + other.m_imag * other.m_imag;
double real = (m_real * other.m_real + m_imag * other.m_imag) / denominator;
double imag = (m_imag * other.m_real - m_real * other.m_imag) / denominator;
return Complex(real, imag);
}
friend std::ostream& operator<<(std::ostream& out, const Complex& c) {
out << c.m_real << " + " << c.m_imag << "i";
return out;
}
private:
double m_real;
double m_imag;
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
Complex c5 = c1 * c2;
Complex c6 = c1 / c2;
std::cout << c3 << std::endl;
std::cout << c4 << std::endl;
std::cout << c5 << std::endl;
std::cout << c6 << std::endl;
return 0;
}
```
运行结果:
```
4 + 6i
-2 - 2i
-5 + 10i
0.44 - 0.08i
```
注意,这里实现的是复数的基本运算,可能还需要根据具体需求扩展其他功能。
相关推荐
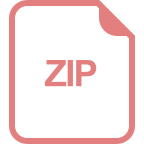














