c++实现复数的加减乘除自加自减
时间: 2023-08-23 07:12:46 浏览: 43
好的,下面是c++实现复数的加减乘除自加自减的代码:
```c++
#include<iostream>
using namespace std;
class Complex{
public:
double real, imag; // 复数的实部和虚部
Complex(double r = 0, double i = 0):real(r), imag(i){}
Complex(const Complex& c){real = c.real; imag = c.imag;}
Complex operator+(const Complex& c2) const { return Complex(real + c2.real, imag + c2.imag); }
Complex operator-(const Complex& c2) const { return Complex(real - c2.real, imag - c2.imag); }
Complex operator*(const Complex& c2) const { return Complex(real * c2.real - imag * c2.imag, real * c2.imag + imag * c2.real); }
Complex operator/(const Complex& c2) const { double d = c2.real * c2.real + c2.imag * c2.imag; return Complex((real * c2.real + imag * c2.imag) / d, (imag * c2.real - real * c2.imag) / d); }
Complex& operator++() { ++real; return *this; }
Complex operator++(int) { Complex t = *this; ++real; return t; }
Complex& operator--() { --real; return *this; }
Complex operator--(int) { Complex t = *this; --real; return t; }
friend ostream& operator<<(ostream& os, const Complex& c){
os << "(" << c.real << "," << c.imag << ")";
return os;
}
};
int main(){
Complex c1(1, 2), c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
cout << "++c1 = " << ++c1 << endl;
cout << "c1++ = " << c1++ << endl;
cout << "--c1 = " << --c1 << endl;
cout << "c1-- = " << c1-- << endl;
return 0;
}
```
这段代码定义了一个Complex类,实现了复数的加减乘除运算和自加自减运算。其中,operator+、operator-、operator*、operator/分别实现了复数的加减乘除运算;operator++、operator--分别实现了复数的自加自减运算;ostream& operator<<重载了输出运算符,用于输出Complex对象的值。在main函数中,我们定义了两个Complex对象c1和c2,并测试了各种运算和自加自减运算的正确性。
相关推荐
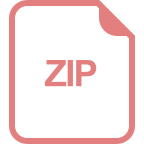














